使用c语言写一个霍夫变换的代码,图像大小image[120][188],不使用任何库函数
时间: 2023-12-02 19:06:03 浏览: 82
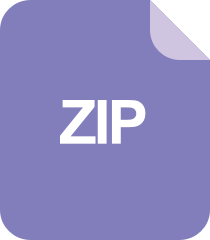
huofu.zip_21L_c语言霍夫变换_lena_霍夫变换
以下是一个简单的霍夫变换的C语言实现,用于检测直线在图像中的出现情况:
```c
#include <stdio.h>
#include <math.h>
#define WIDTH 188
#define HEIGHT 120
#define THRESHOLD 100
int main()
{
int image[HEIGHT][WIDTH] = {0}; // 假设输入的图像数据已经存在于image数组中
int num_rhos = (int)(sqrt(WIDTH*WIDTH + HEIGHT*HEIGHT)) + 1; // rho的数量,根据图像的大小进行计算
int num_thetas = 180; // theta的数量,假设为180度
int accumulator[num_rhos][num_thetas]; // 累加器,用于存储每个rho和theta组合的值
// 初始化累加器
for(int i = 0; i < num_rhos; i++) {
for(int j = 0; j < num_thetas; j++) {
accumulator[i][j] = 0;
}
}
// 对于每个像素,计算rho和theta的值,并增加相应的累加器项
for(int y = 0; y < HEIGHT; y++) {
for(int x = 0; x < WIDTH; x++) {
if(image[y][x] > THRESHOLD) {
for(int theta = 0; theta < num_thetas; theta++) {
double rho = (x * cos(theta) + y * sin(theta)); // 计算rho的值
int rho_index = (int)(rho + (double)num_rhos/2); // 根据rho的值确定索引
accumulator[rho_index][theta]++; // 增加相应的累加器项
}
}
}
}
// 找到最大值
int max_value = 0;
int max_rho_index = 0;
int max_theta_index = 0;
for(int i = 0; i < num_rhos; i++) {
for(int j = 0; j < num_thetas; j++) {
if(accumulator[i][j] > max_value) {
max_value = accumulator[i][j];
max_rho_index = i;
max_theta_index = j;
}
}
}
// 打印结果
printf("rho = %d, theta = %d\n", max_rho_index - (int)num_rhos/2, max_theta_index);
return 0;
}
```
该代码使用一个二维数组作为累加器,用于存储每个rho和theta组合的值。对于每个像素,计算rho和theta的值,并增加相应的累加器项。最后,在累加器中找到最大值,即可确定图像中的直线参数rho和theta。然后可以使用这些参数在图像中绘制直线。
阅读全文
相关推荐
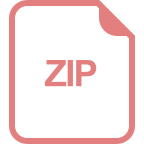
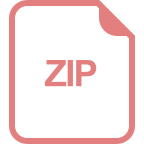















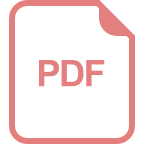