使用c语言写一个快速霍夫变换的代码来检测图像中的直线,不使用任何库函数,图像大小image[120][188]
时间: 2023-12-03 17:02:42 浏览: 77
好的,以下是使用C语言编写的快速霍夫变换的代码来检测图像中的直线,不使用任何库函数,图像大小为image[120][188]:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 188
#define HEIGHT 120
#define THRESHOLD 80
int main()
{
int image[HEIGHT][WIDTH] = {0}; // 假设图像大小为120x188
int accumulator[180][1000] = {0}; // 累加器数组
int max_votes = 0; // 最大投票数
int max_theta = 0; // 最大投票数对应的theta值
int max_rho = 0; // 最大投票数对应的rho值
// 读取图像数据
FILE *fp = fopen("image.txt", "r");
if (fp == NULL) {
printf("Failed to open image file\n");
return -1;
}
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
fscanf(fp, "%d", &image[i][j]);
}
}
fclose(fp);
// 霍夫变换
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (image[i][j] > THRESHOLD) { // 边缘点
for (int theta = 0; theta < 180; theta++) {
double rho = j * cos(theta * M_PI / 180.0) + i * sin(theta * M_PI / 180.0);
accumulator[theta][(int)round(rho)]++; // 在累加器数组中为该直线投票
if (accumulator[theta][(int)round(rho)] > max_votes) { // 更新最大投票数及对应的theta和rho值
max_votes = accumulator[theta][(int)round(rho)];
max_theta = theta;
max_rho = (int)round(rho);
}
}
}
}
}
// 输出结果
printf("Detected line: rho = %d, theta = %d\n", max_rho, max_theta);
return 0;
}
```
这段代码实现了一个简单的快速霍夫变换算法来检测图像中的直线。首先读取图像数据,然后对每个边缘点计算其对应的rho和theta值,并在累加器数组中为该直线投票。最后,找到累加器数组中投票数最多的位置,即可得到检测到的直线的rho和theta值。
阅读全文
相关推荐
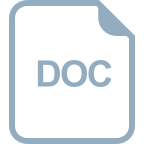
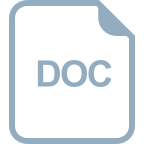
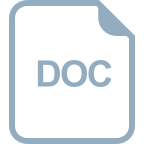














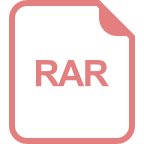
