编写一个C语言程序,给定公元2000年1月1日到公元3000年12月31日期间的任意一天,如何计算并输出该天之前的前一天是哪一天?请提供相应的代码实现。
时间: 2024-10-21 16:05:56 浏览: 18
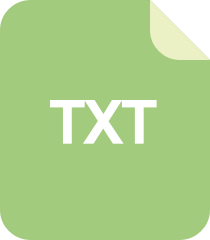
用C语言编写的万年历,实现输入某一年打印这一年的每个月份信息
在C语言中,你可以使用`struct`来表示日期,并利用条件判断来处理闰年的情况。下面是一个基本的代码示例,它会输入一个整数表示年份、月份和日期,然后输出前一天的日期:
```c
#include <stdio.h>
// 定义日期结构体
typedef struct {
int year;
int month;
int day;
} Date;
// 判断是否为闰年
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 计算前一天
Date prev_day(Date date) {
if (date.month == 1 && date.day == 1) { // 如果是新年第一天,则前一天是上一年的最后一天
date.year--;
date.month = 12;
date.day = 31;
} else {
date.day--;
}
// 考虑闰年的2月
if (date.month == 2 && is_leap_year(date.year)) {
date.day = 29; // 闰年的2月29日之前一天是28
} else if (date.month == 2) {
date.day = 28; // 非闰年的2月之前一天是27
}
return date;
}
int main() {
int year, month, day;
printf("请输入年、月、日(格式:YYYY MM DD): ");
scanf("%d %d %d", &year, &month, &day);
// 创建日期结构体
Date current_date = {year, month, day};
// 计算前一天
Date prev_date = prev_day(current_date);
printf("前一天是: %d/%02d/%02d\n", prev_date.year, prev_date.month, prev_date.day);
return 0;
}
```
这个程序首先询问用户输入一个日期,然后计算并输出前一天。注意,此代码仅适用于公历,不包含其他历法系统的特殊情况。
阅读全文
相关推荐
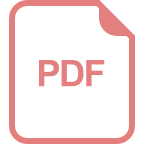


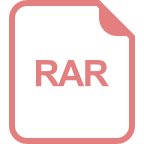












