迷宫问题c语言实现代码
时间: 2024-06-23 08:01:34 浏览: 193
迷宫问题在C语言中通常可以通过广度优先搜索(BFS)或深度优先搜索(DFS)算法来解决。这里我会提供一个简单的二维迷宫遍历的BFS解法示例。请注意,这只是一个基础版本,实际的迷宫可能需要处理边界条件、死路等复杂情况。
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define ROWS 5 // 迷宫行数
#define COLS 7 // 迷宫列数
#define WALL '#'
// 二维数组表示迷宫
char maze[ROWS][COLS] = {
{'#', '#', '#', '#', '#', '#', '#'},
{'#', ' ', ' ', '.', ' ', '#', '#'},
{'#', ' ', '.', ' ', '.', ' ', '#'},
{'#', ' ', '.', ' ', '.', ' ', '#'},
{'#', '#', '#', '#', '#', '#', '#'}
};
// 用于存储路径的辅助数组
bool visited[ROWS][COLS];
// 遍历迷宫的函数
void bfs(int start_row, int start_col) {
int rows = ROWS;
int cols = COLS;
queue<int> q;
// 设置起点为已访问
visited[start_row][start_col] = true;
q.push(start_row * cols + start_col);
while (!q.empty()) {
int row = q.front() / cols;
int col = q.front() % cols;
q.pop();
printf("(%d,%d) ", row, col); // 打印路径
// 检查上下左右邻居是否合法并未访问
if (row > 0 && maze[row-1][col] = true;
q.push((row-1) * cols + col);
}
if (row < rows-1 && maze[row+1][col] != WALL && !visited[row+1][col]) {
visited[row+1][col] = true;
q.push((row+1) * cols + col);
}
if (col > 0 && maze[row][col-1] != WALL && !visited[row][col-1]) {
visited[row][col-1] = true;
q.push(row * cols + col - 1);
}
if (col < cols-1 && maze[row][col+1] != WALL && !visited[row][col+1]) {
visited[row][col+1] = true;
q.push(row * cols + col + 1);
}
}
}
int main() {
bfs(1, 3); // 从起始位置 (1,3) 开始遍历
printf("\nEnd of path.\n");
return 0;
}
```
阅读全文
相关推荐
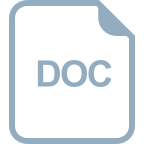
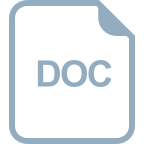
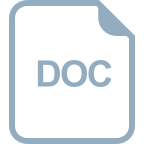


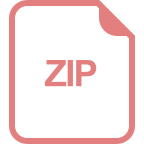
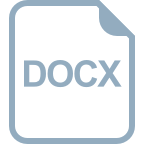
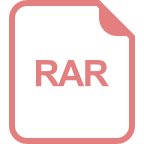
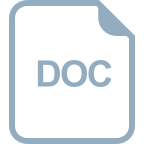






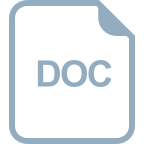
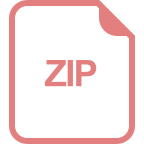