Transformers实战
时间: 2023-11-14 07:06:40 浏览: 49
好的,我了解了你的要求。让我来回答你关于Transformers的问题。
Transformers是一种基于自注意力机制的深度学习模型,它在自然语言处理领域取得了很大的成功。它的核心思想是利用自注意力机制来处理输入序列中每个元素的关系,而不需要利用循环神经网络或卷积神经网络。
在实战中,Transformers可以用于各种自然语言处理任务,包括文本分类、命名实体识别、机器翻译等。为了使用Transformers,我们通常需要进行以下步骤:
1. 数据预处理:将原始文本数据转换为模型可接受的格式,包括分词、编码等操作。
2. 模型构建:选择合适的Transformers模型,例如BERT、GPT等,并根据任务需求进行相应的修改和调整。
3. 模型训练:使用预处理后的数据对模型进行训练,并根据任务需求选择合适的损失函数和优化算法。
4. 模型评估:使用测试集对训练好的模型进行评估,通常使用准确率、F1值等指标来衡量模型性能。
5. 模型应用:将训练好的模型用于实际应用中,可以进行文本分类、文本生成等各种任务。
相关问题
huggingface transformers实战
Hugging Face Transformers 是一个基于 PyTorch 和 TensorFlow 的自然语言处理(NLP)库,它提供了用于训练、微调和使用最先进的预训练模型的工具和接口。以下是使用 Hugging Face Transformers 进行实战的一些示例。
1. 文本分类
文本分类是将文本分为不同的类别或标签的任务。在这个示例中,我们将使用 Hugging Face Transformers 中的 DistilBERT 模型来训练一个情感分析分类器,以将电影评论分为正面或负面。
```python
from transformers import DistilBertTokenizer, DistilBertForSequenceClassification
import torch
tokenizer = DistilBertTokenizer.from_pretrained('distilbert-base-uncased')
model = DistilBertForSequenceClassification.from_pretrained('distilbert-base-uncased')
# 训练数据
train_texts = ["I really liked this movie", "The plot was boring and predictable"]
train_labels = [1, 0]
# 将文本编码为输入张量
train_encodings = tokenizer(train_texts, truncation=True, padding=True)
# 将标签编码为张量
train_labels = torch.tensor(train_labels)
# 训练模型
model.train()
optimizer = torch.optim.AdamW(model.parameters(), lr=5e-5)
for epoch in range(3):
optimizer.zero_grad()
outputs = model(**train_encodings, labels=train_labels)
loss = outputs.loss
loss.backward()
optimizer.step()
# 预测新的评论
texts = ["This is a great movie", "I hated this movie"]
encodings = tokenizer(texts, truncation=True, padding=True)
model.eval()
with torch.no_grad():
outputs = model(**encodings)
predictions = torch.argmax(outputs.logits, dim=1)
print(predictions)
```
2. 问答系统
问答系统是回答用户提出的问题的模型。在这个示例中,我们将使用 Hugging Face Transformers 中的 DistilBERT 模型和 SQuAD 数据集来训练一个简单的问答系统。
```python
from transformers import DistilBertTokenizer, DistilBertForQuestionAnswering
import torch
tokenizer = DistilBertTokenizer.from_pretrained('distilbert-base-uncased')
model = DistilBertForQuestionAnswering.from_pretrained('distilbert-base-uncased')
# 加载 SQuAD 数据集
from transformers import squad_convert_examples_to_features, SquadExample, SquadFeatures, squad_processors
processor = squad_processors['squad']
examples = processor.get_train_examples('data')
features = squad_convert_examples_to_features(examples=examples, tokenizer=tokenizer, max_seq_length=384, doc_stride=128, max_query_length=64, is_training=True)
# 训练模型
model.train()
optimizer = torch.optim.AdamW(model.parameters(), lr=5e-5)
for epoch in range(3):
for feature in features:
optimizer.zero_grad()
outputs = model(input_ids=torch.tensor([feature.input_ids]), attention_mask=torch.tensor([feature.attention_mask]), start_positions=torch.tensor([feature.start_position]), end_positions=torch.tensor([feature.end_position]))
loss = outputs.loss
loss.backward()
optimizer.step()
# 预测新的问题
text = "What is the capital of France?"
question = "What country's capital is Paris?"
inputs = tokenizer.encode_plus(question, text, add_special_tokens=True, return_tensors='pt')
model.eval()
with torch.no_grad():
start_scores, end_scores = model(**inputs)
start_index = torch.argmax(start_scores)
end_index = torch.argmax(end_scores)
answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(inputs['input_ids'][0][start_index:end_index+1]))
print(answer)
```
3. 文本生成
文本生成是使用预训练模型生成自然语言文本的任务。在这个示例中,我们将使用 Hugging Face Transformers 中的 GPT-2 模型生成一些小说的开头。
```python
from transformers import GPT2Tokenizer, GPT2LMHeadModel
import torch
tokenizer = GPT2Tokenizer.from_pretrained('gpt2')
model = GPT2LMHeadModel.from_pretrained('gpt2')
# 生成新的文本
seed_text = "In a hole in the ground there lived a hobbit."
encoded = tokenizer.encode(seed_text, return_tensors='pt')
model.eval()
with torch.no_grad():
output = model.generate(encoded, max_length=100, do_sample=True)
generated = tokenizer.decode(output[0], skip_special_tokens=True)
print(generated)
```
这些示例只是 Hugging Face Transformers 库的一部分功能。您可以通过访问 Hugging Face Transformers 官方文档来了解更多信息。
transformers教程
以下是一些学习transformers的教程和资源:
1. Transformers官方文档:https://huggingface.co/transformers/
2. The Illustrated Transformer:一篇图文并茂的transformer解释文章,非常易懂:http://jalammar.github.io/illustrated-transformer/
3. The Annotated Transformer:一篇注释版的transformer论文,对于理解transformer非常有帮助:http://nlp.seas.harvard.edu/2018/04/03/attention.html
4. Attention Is All You Need(原始transformer论文):https://arxiv.org/abs/1706.03762
5. 《深入浅出Transformer》一书,作者是李宏毅,详细介绍了transformer的原理、实现和应用:https://www.gitbook.com/book/hankcs/transformer/details
6. 《动手学深度学习》一书,其中包含了transformer的实现和应用案例,非常适合入门:https://zh.d2l.ai/
7. Transformers实战教程:https://huggingface.co/course/chapter1
以上是一些学习transformers的教程和资源,可以根据自己的需求和兴趣进行选择和学习。
相关推荐
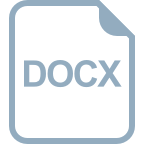
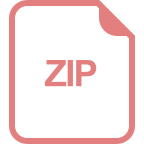
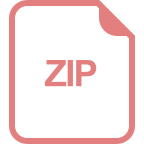












