编写一个C 程序,并使用系统调用fork()创建一个子进程
时间: 2024-06-13 20:04:46 浏览: 361
编写一个C程序,使用系统调用fork()创建一个子进程的示例代码如下:
```
#include <stdio.h>
#include <unistd.h>
int main() {
pid_t pid;
pid = fork();
if (pid < 0) {
fprintf(stderr, "Fork failed");
return 1;
} else if (pid == 0) {
printf("This is the child process\n");
} else {
printf("This is the parent process\n");
}
return 0;
}
```
在这个程序中,我们使用了`fork()`函数来创建一个子进程。`fork()`函数会返回两次,一次在父进程中返回子进程的PID,一次在子进程中返回0。因此,我们可以通过判断`fork()`函数的返回值来确定当前进程是父进程还是子进程。
在这个示例程序中,如果`fork()`函数返回的值小于0,则表示创建子进程失败。如果返回值等于0,则表示当前进程是子进程,我们在子进程中输出一条信息。如果返回值大于0,则表示当前进程是父进程,我们在父进程中输出一条信息。
相关问题
编写一个程序使用系统调用fork生成3个子进程并使用系统调用pipc创建一个管道,使得
编写一个程序使用系统调用fork生成3个子进程并使用系统调用pipc创建一个管道,使得子进程之间可以通过管道进行通信。
首先,需要包含头文件<sys/types.h>、<sys/stat.h>、<fcntl.h>、<unistd.h>和<stdio.h>来使用相关的系统调用。
程序的主函数如下所示:
```c
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <stdio.h>
int main() {
pid_t pid[3];
int fd[2];
// 创建管道
if (pipe(fd) < 0) {
perror("创建管道失败");
return 1;
}
// 创建子进程
for (int i = 0; i < 3; i++) {
pid[i] = fork();
if (pid[i] < 0) {
perror("创建子进程失败");
return 1;
} else if (pid[i] == 0) {
// 子进程从管道中读取数据
close(fd[1]); // 关闭写端
char buffer[256];
read(fd[0], buffer, sizeof(buffer));
printf("子进程%d收到的数据:%s\n", i + 1, buffer);
return 0;
}
}
// 父进程向管道中写入数据
close(fd[0]); // 关闭读端
char message[] = "Hello, child processes!";
write(fd[1], message, sizeof(message));
return 0;
}
```
在程序中,首先使用系统调用pipe(fd)创建一个管道,fd[0]表示读端,fd[1]表示写端。然后使用for循环创建3个子进程,并在子进程中使用read系统调用从管道中读取数据,并输出接收到的消息。最后,在父进程中使用write系统调用向管道中写入数据。
需要注意的是,在父进程中,需要通过close系统调用关闭不使用的文件描述符,这里关闭了管道的读端fd[0],以及在子进程中关闭了管道的写端fd[1]。
这样,运行程序后,父进程向管道中写入数据,然后每个子进程从管道中读取数据并输出,实现了子进程之间通过管道进行通信。
编写一个C程序,并使用系统调用fork()创建一个子进程。要求如下: (1)在子进程中分别输入当前进程为子进程单位提示,当前进程的PID 和父进程的pid,根据用户输入确定当前进程的返回值,退出信息等消息。 (2)编写另一个C程序,使用系统调用fork()以创建一个子进程,并使用这个子进程调用exec函数族以执行系统命令ls。
您好,欢迎使用ChitGPT进行对话。根据您的要求,我可以为您提供以下C代码:
```
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/wait.h>
int main()
{
//使用fork()创建子进程
pid_t pid = fork();
if (pid < 0) {
//创建子进程失败
fprintf(stderr, "创建子进程失败!\n");
return 1;
} else if (pid == 0) {
//在子进程中执行以下操作
printf("当前进程为子进程,PID为%d,父进程的pid为%d\n",
getpid(), getppid());
//根据用户输入确定当前进程的返回值和退出信息
int r_value;
printf("请输入当前进程的返回值:");
scanf("%d", &r_value);
return r_value;
} else {
//在父进程中执行以下操作
printf("当前进程为父进程,PID为%d,子进程的pid为%d\n",
getpid(), pid);
//等待子进程退出并获取其退出状态
int status;
wait(&status);
if (WIFEXITED(status)) {
printf("子进程返回值为%d\n", WEXITSTATUS(status));
} else {
printf("子进程异常退出!\n");
}
}
return 0;
}
```
另一个C程序如下:
```
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/wait.h>
int main()
{
//使用fork()创建子进程
pid_t pid = fork();
if (pid < 0) {
//创建子进程失败
fprintf(stderr, "创建子进程失败!\n");
return 1;
} else if (pid == 0) {
//在子进程中执行以下操作
execl("/bin/ls", "ls", NULL);
} else {
//在父进程中等待子进程退出
wait(NULL);
printf("子进程已结束!\n");
}
return 0;
}
```
希望这些代码能够帮助您解决问题。如果您有其他问题或者需要更多的帮助,请随时告诉我。
阅读全文
相关推荐
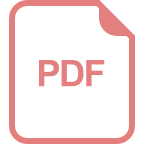
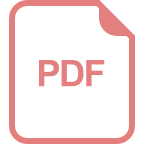
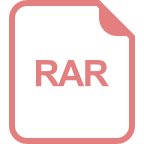













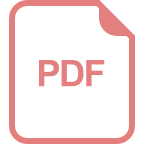
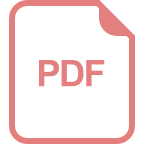