用python实现遗传算法求解下列y=x*sin(x)最小值
时间: 2024-12-22 12:19:11 浏览: 8
在Python中,我们可以使用遗传算法(Genetic Algorithm, GA)来解决优化问题,例如寻找函数`y = x * sin(x)`的最小值。遗传算法是一种模拟自然选择过程的搜索技术,它通过迭代的方式生成新的解,并通过适应度函数评估优劣,然后选择最好的个体进行“繁殖”,以此寻找全局最优解。
以下是简单的步骤:
1. **初始化种群**:随机生成一组初始解(也称作染色体),每个解代表一个可能的参数x。
2. **计算适应度**:对每个解,计算其对应的函数值`f(x) = x * sin(x)`,适应度通常是该函数值的负数,因为我们要找的是最小值。
3. **选择操作**:根据每个个体的适应度,通常采用轮盘赌选择法或锦标赛选择法,选取一部分优秀的个体进入下一轮。
4. **交叉操作**:对选出的部分个体进行配对并进行交叉,即交换两个个体的一部分基因(这里就是x值)。
5. **变异操作**:对产生的新个体进行一些随机变化,以增加种群多样性,防止陷入局部最优。
6. **重复步骤**:直到达到预设的最大代数次数,或者适应度函数的变化足够小。
7. **返回最佳解**:最后得到的适应度最高的个体就是所求的解。
下面是一个简化版的遗传算法实现示例:
```python
import random
import numpy as np
def fitness(chromosome):
x = chromosome
return -np.sin(x)
def selection(population, fitness_scores):
# 轮盘赌选择
total_fitness = sum(fitness_scores)
probabilities = [score / total_fitness for score in fitness_scores]
return random.choices(population, probabilities, k=len(population))
def crossover(parents):
# 单点交叉
parent1, parent2 = parents
crossover_point = random.randint(0, len(parent1))
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
def mutation(child):
# 变异
mutated_child = list(child)
if random.random() < 0.1: # 随机变异概率
mutated_child[random.randint(0, len(mutated_child)-1)] += random.uniform(-1, 1)
return tuple(mutated_child)
# 初始化种群和适应度
pop_size = 50
population = [(random.uniform(-10, 10),) for _ in range(pop_size)]
fitness_scores = [-fitness(chromosome[0]) for chromosome in population]
for generation in range(max_gens): # 迭代次数
new_population = []
selected_pop = selection(population, fitness_scores)
while len(new_population) < pop_size:
parent1, parent2 = random.sample(selected_pop, 2)
child1, child2 = crossover((parent1, parent2))
child1 = mutation(child1)
child2 = mutation(child2)
new_population.extend([child1, child2])
population = new_population
# 更新适应度,这里只取第一个元素作为解
fitness_scores = [-fitness(chromosome[0]) for chromosome in population]
best_solution = population[np.argmax(fitness_scores)]
print("Best solution found: x =", best_solution[0], "with y =", best_solution[0] * np.sin(best_solution[0]))
```
阅读全文
相关推荐
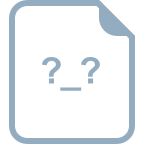
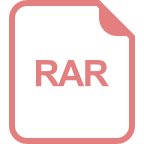
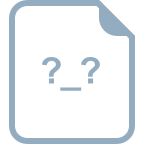










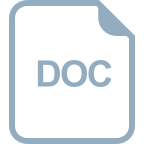




