请你用c++实现功能:删除某个路径下的所有图片
时间: 2024-10-28 21:12:20 浏览: 10
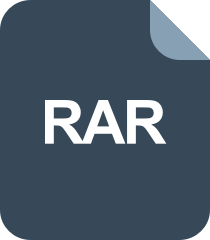
c++删除某个路径下的所有文件(源代码和程序)
在C++中,要实现删除指定路径下所有图片的功能,通常我们会使用文件操作和目录遍历的相关库,如`<filesystem>`头文件提供的API。这里是一个简单的示例,假设我们有权限访问文件系统并能删除文件:
```cpp
#include <iostream>
#include <filesystem>
bool deleteFilesInDir(const std::string& dirPath) {
try {
for (const auto& entry : filesystem::directory_iterator(dirPath)) {
if (entry.is_regular_file() && entry.path().has_extension(".jpg") || entry.path().has_extension(".png") || // 这里假设图片常见格式是.jpg和.png
entry.path().has_extension(".bmp") || entry.path().has_extension(".gif")) { // 可以添加更多图片格式
std::cout << "Deleting file: " << entry.path().string() << std::endl;
filesystem::remove(entry.path()); // 删除文件
}
}
return true;
} catch (const filesystem::filesystem_error& e) {
std::cerr << "Error deleting files: " << e.what() << std::endl;
return false;
}
}
int main() {
const std::string directoryToClear = "path_to_your_directory"; // 替换为你想删除图片的实际路径
if (deleteFilesInDir(directoryToClear)) {
std::cout << "All images in the directory have been deleted." << std::endl;
} else {
std::cout << "Deletion failed." << std::endl;
}
return 0;
}
```
注意:
1. 这段代码需要C++17或更高版本的支持,因为`<filesystem>`库是在C++17引入的。
2. 删除文件可能会有权限限制,确保程序有足够的权限执行此操作。
3. 使用`filesystem::remove`函数时需谨慎,因为它会永久删除文件。
阅读全文
相关推荐
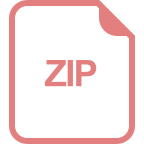
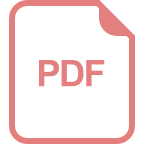
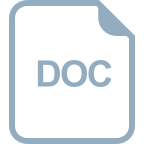
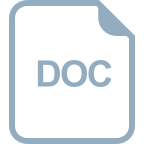
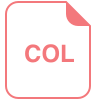
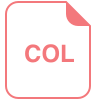
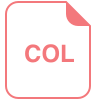

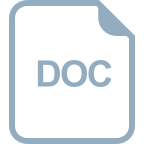
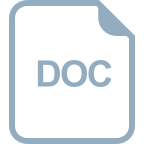
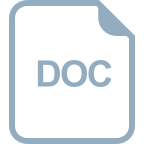
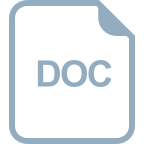
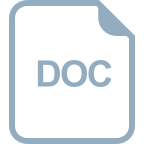
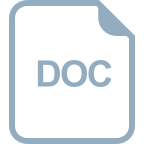
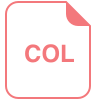
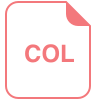
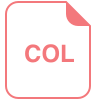
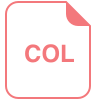