用结构体描述一个整形类型的单链表。创建线性表,在该线性表中实现插入元素、删除元素、查找等操作。设计选择式菜单,以选择菜单方式进行操作。
时间: 2024-12-13 09:20:53 浏览: 10
在C语言中,我们可以使用结构体(struct)来描述一个整型类型的单链表。首先,我们需要定义一个结构体`ListNode`,包含整数值`value`和指向下一个节点的指针`next`:
```c
typedef struct ListNode {
int value;
struct ListNode* next;
} ListNode;
```
接下来,我们可以创建相关的链表操作函数:
1. **创建链表**:初始化一个空链表
```c
ListNode* createList() {
return NULL;
}
```
2. **插入元素**:在链表尾部添加一个新节点
```c
void insertNode(ListNode* list, int value) {
if (list == NULL) {
list = (ListNode*)malloc(sizeof(ListNode));
list->value = value;
list->next = NULL;
} else {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->value = value;
newNode->next = list->next;
list->next = newNode;
}
}
```
3. **删除元素**:根据值删除指定位置的节点
```c
void deleteNode(ListNode** list, int value) {
ListNode* current = *list, *prev = NULL;
while (current != NULL && current->value != value) {
prev = current;
current = current->next;
}
if (current != NULL) {
if (prev == NULL) {
*list = current->next; // 删除头节点
} else {
prev->next = current->next;
}
free(current);
}
}
```
4. **查找元素**:遍历链表找到目标值的位置
```c
int searchNode(ListNode* list, int value) {
ListNode* current = list;
while (current != NULL) {
if (current->value == value) {
return 1; // 找到,返回1表示存在
}
current = current->next;
}
return 0; // 没有找到,返回0
}
```
为了实现选择式菜单,你可以编写一个循环,让用户输入选项并根据输入调用相应的函数:
```c
int main() {
ListNode* list = createList();
while (1) {
printf("请选择操作:\n");
printf("1. 插入\n2. 删除\n3. 查找\n4. 退出\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 1:
int value;
printf("请输入要插入的值: ");
scanf("%d", &value);
insertNode(&list, value);
break;
case 2:
int targetValue;
printf("请输入要删除的值: ");
scanf("%d", &targetValue);
deleteNode(&list, targetValue);
break;
case 3:
int targetFind;
printf("请输入要查找的值: ");
scanf("%d", &targetFind);
if (searchNode(list, targetFind)) {
printf("找到该值\n");
} else {
printf("未找到该值\n");
}
break;
case 4:
printf("程序结束.\n");
exit(0);
default:
printf("无效的选择, 请重新输入.\n");
break;
}
}
return 0;
}
阅读全文
相关推荐
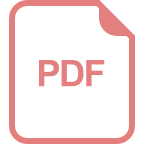
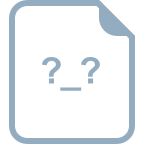
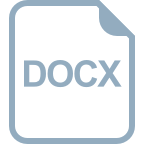


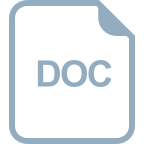










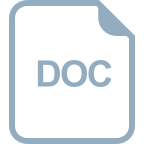
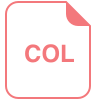
