用结构体描述一个整形类型的单链表。创建线性表,在该线性表中实现插入元素、删除元素、查找等操作。设计选择式菜单,以选择菜单方式进行操作。用EDV编写
时间: 2024-12-13 09:23:34 浏览: 7
在C语言中,可以使用结构体(struct)来描述一个整型类型的单链表。首先,我们定义一个节点结构体`ListNode`:
```c
typedef struct ListNode {
int data; // 节点数据
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
```
接下来,我们可以实现一些基本操作,如插入元素、删除元素和查找:
1. **插入元素**:
```c
void insertNode(ListNode** head, int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
```
2. **删除元素**:
```c
void deleteNode(ListNode** head, int value) {
if (*head == NULL) return;
if ((*head)->data == value) {
ListNode* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
ListNode* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
ListNode* toDelete = current->next;
current->next = current->next->next;
free(toDelete);
}
}
```
3. **查找元素**:
```c
ListNode* searchNode(ListNode* head, int value) {
ListNode* current = head;
while (current != NULL) {
if (current->data == value) return current;
current = current->next;
}
return NULL;
}
```
为了提供用户选择操作的功能,你可以设计一个简单的菜单驱动程序:
```c
int main() {
ListNode* head = NULL;
char choice;
do {
printf("\n1. 插入元素\n2. 删除元素\n3. 查找元素\n4. 退出\n");
scanf("%d", &choice);
switch(choice) {
case 1:
int value;
printf("请输入值:");
scanf("%d", &value);
insertNode(&head, value);
break;
case 2:
int valueToDelete;
printf("请输入要删除的值:");
scanf("%d", &valueToDelete);
deleteNode(&head, valueToDelete);
break;
case 3:
int searchValue;
printf("请输入查找的值:");
scanf("%d", &searchValue);
ListNode* found = searchNode(head, searchValue);
if (found) printf("找到值 %d\n", found->data);
else printf("未找到\n");
break;
case 4:
printf("退出程序...\n");
break;
default:
printf("无效选择,请重新输入。\n");
}
} while (choice != 4);
return 0;
}
```
在这个例子中,用户可以选择操作并按照提示执行相应的功能。
阅读全文
相关推荐
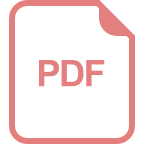
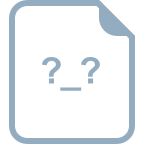
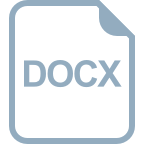


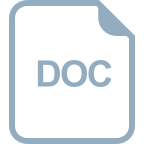










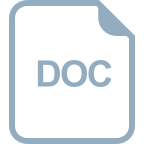
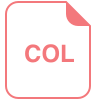
