后缀为csv的波士顿房价数据文件存放在文件夹路径csv_file_dir中。按下列考试要求进行数据处理: 1.读取数据文件中的所有数据为DataFrame格式,保留第0行的表头作为列名。获得样本列名为y_target列赋值给y,除此之外的13列赋值给X; 2.使用sklearn中的sklearn.feature_selection.VarianceThreshold定义基于方差的筛选模型,方差阈值threshold设置为10,其他参数保持默认值; 3.使用fit_transform训练2定义的筛选模型返回选出的新的特征X_new; 4.将第3步得到的特征数据X_new与y按列合并处理成新的DataFrame,按student_answer_path生成csv文件并保存,编码方式采用‘UTF-8’,所有值保留3位小数,小数点后尾部的0无需保存,如:0.200直接保存成0.2,不保留列名及行索引。 提示 df = pd.read_csv(filepath,header) # filepath相对路径,header默认为0,header=None时,表头读为表的信息不做列名 sklearn.feature_selection.VarianceThreshold(threshold) # 定义筛选模型 fit_transform(X, y) # 训练模型 np.concatenate((arr1, arr2), axis=1) # ndarray 拼接 np.round(x, 3) # 对x保留3位小数 df.to_csv(savepath, index=False, encoding='UTF-8') # index参数表示保存为.csv文件是否保留index 输出示例 0.00632,18.0,2.31,65.2,1.0,296.0,396.9,4.98,24.0 0.02731,0.0,7.07,78.9,2.0,242.0,396.9,9.14,21.6 0.02729,0.0,7.07,61.1,2.0,242.0,392.83,4.03,34.7
时间: 2024-02-27 14:56:10 浏览: 23
以下是实现该考试要求的代码:
```python
import pandas as pd
import numpy as np
from sklearn.feature_selection import VarianceThreshold
# 读取数据文件
csv_file_dir = 'path/to/csv_file_dir'
df = pd.read_csv(csv_file_dir, header=0)
# 分离X和y
y = df['y_target']
X = df.drop('y_target', axis=1)
# 定义基于方差的筛选模型
threshold = 10
variance_selector = VarianceThreshold(threshold)
# 训练筛选模型并得到新的特征X_new
X_new = variance_selector.fit_transform(X)
# 将X_new和y按列合并成新的DataFrame
merged_df = pd.DataFrame(np.concatenate((X_new, y.values.reshape(-1, 1)), axis=1))
# 将结果保存到csv文件中
student_answer_path = 'path/to/student_answer.csv'
merged_df.to_csv(student_answer_path, index=False, encoding='UTF-8', header=False, float_format='%.3f')
```
注意:在保存结果到csv文件时,需要将header参数设置为False以不保存列名,将float_format参数设置为'%.3f'以保留3位小数。
相关推荐
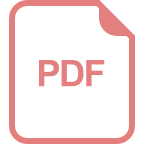
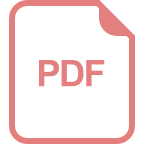















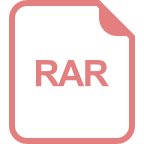