请你实现一个循环队列,该循环队列可利用的空间大小等于n个int型变量的大小。 操作: push x:将x加入到循环队列尾端。若循环队列已满,输出"full"(不含引号),否则不输出任何内容。保证x为int型整数。 front:输出队首元素,队首不出队。若队列为空,输出"empty"(不含引号)。 pop:输出队首元素,且队首出队。若队列为空,输出"empty"(不含引号)。 第一行输入两个整数n,q (1≤n,q≤105),表示循环队列可利用的空间大小和操作次数。 接下来的q行,每行一个字符串,表示一个
时间: 2024-02-13 11:05:22 浏览: 101
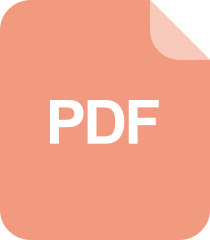
如何实现循环队列
操作。如果是 push 操作,后面会跟随一个整数x。如果是 front 和 pop 操作,则后面没有任何参数。
以下是一个可能的实现,采用双指针来维护循环队列。其中,front指针指向队首元素,rear指针指向队尾元素的下一个位置。当front和rear指向同一位置时,表示队列为空;当rear的下一个位置是front时,表示队列已满。在 push 操作时,如果队列已满,则不执行任何操作。在 pop 和 front 操作时,如果队列为空,则输出 "empty"。
```
#include <iostream>
using namespace std;
int n, q;
int queue[100005];
int front = 0, rear = 0;
void push(int x) {
if ((rear+1)%n == front) {
cout << "full" << endl;
} else {
queue[rear] = x;
rear = (rear+1)%n;
}
}
void pop() {
if (front == rear) {
cout << "empty" << endl;
} else {
cout << queue[front] << endl;
front = (front+1)%n;
}
}
void front_element() {
if (front == rear) {
cout << "empty" << endl;
} else {
cout << queue[front] << endl;
}
}
int main() {
cin >> n >> q;
while (q--) {
string op;
cin >> op;
if (op == "push") {
int x;
cin >> x;
push(x);
} else if (op == "pop") {
pop();
} else if (op == "front") {
front_element();
}
}
return 0;
}
```
阅读全文
相关推荐
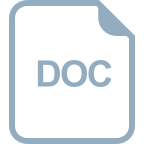














