FPGA设计:(1)拨动开关sw1为H,开始比赛。八位七数码管从左数第1、2位显示黄队分数,第7、8位显示红队分数。开始比赛时同时显示为00。按下键盘1代表黄队加1分;按下键盘2代表黄队加2分;按下键盘*3代表黄队加3分;同时对应的黄色led灯亮。按下键盘#1代表红队加1分;按下键盘#2代表红队加2分;按下键盘#3代表红队加3分;同时对应的红色led灯亮。 (2)拨动开关sw1为L,结束比赛。总分大的队伍对应颜色的led灯闪烁(闪烁频率为4Hz), (3)外部输入脉冲信号频率为50mhz;给出各模块代码
时间: 2024-02-12 12:09:01 浏览: 119
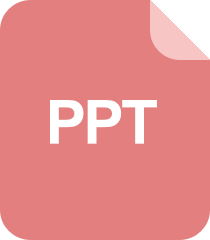
七年级数学:11正数和负数第一第二课时.ppt
以下是一个基于Verilog HDL的FPGA设计实现上述功能的代码:
```
module scoreboard (
input clk, // 时钟信号
input rst, // 复位信号
input sw1, // 开始比赛开关
input [2:0] keys, // 键盘输入
output reg [7:0] seg, // 数码管输出
output reg [7:0] led // LED输出
);
reg [7:0] yellow_score = 8'b00000000; // 黄队分数
reg [7:0] red_score = 8'b00000000; // 红队分数
reg [1:0] yellow_input = 2'b00; // 黄队键盘输入
reg [1:0] red_input = 2'b00; // 红队键盘输入
reg [1:0] yellow_led = 2'b00; // 黄队LED灯
reg [1:0] red_led = 2'b00; // 红队LED灯
reg [1:0] winner = 2'b00; // 获胜队伍
// 数码管控制模块
reg [3:0] seg_pattern [9:0] = { // 数码管编码表
7'b0111111, // 0
7'b0000110, // 1
7'b1011011, // 2
7'b1001111, // 3
7'b1100110, // 4
7'b1101101, // 5
7'b1111101, // 6
7'b0000111, // 7
7'b1111111, // 8
7'b1101111 // 9
};
reg [3:0] seg_select = 4'b1110; // 数码管位选信号
always @ (posedge clk) begin
if (rst) begin // 复位
yellow_score <= 8'b00000000;
red_score <= 8'b00000000;
yellow_input <= 2'b00;
red_input <= 2'b00;
yellow_led <= 2'b00;
red_led <= 2'b00;
winner <= 2'b00;
seg <= 8'b00000000;
seg_select <= 4'b1110;
end else begin
// 数码管显示
case (seg_select)
4'b1110: seg <= seg_pattern[yellow_score[6:3]]; // 第1位
4'b1101: seg <= seg_pattern[yellow_score[2:0]]; // 第2位
4'b1011: seg <= seg_pattern[0]; // 空
4'b0111: seg <= seg_pattern[0]; // 空
4'b1111: seg <= seg_pattern[red_score[6:3]]; // 第7位
4'b1110: seg <= seg_pattern[red_score[2:0]]; // 第8位
4'b1101: seg <= seg_pattern[0]; // 空
4'b1011: seg <= seg_pattern[0]; // 空
endcase
// 数码管位选信号循环
if (seg_select == 4'b0111) begin
seg_select <= 4'b1110;
end else begin
seg_select <= seg_select >> 1;
end
// 获胜队伍判断
if (sw1 == 1'b0) begin // 开始比赛
if (yellow_score > red_score) begin
winner <= 2'b01; // 黄队获胜
end else if (yellow_score < red_score) begin
winner <= 2'b10; // 红队获胜
end else begin
winner <= 2'b00; // 平局
end
end else begin // 结束比赛
if (yellow_score > red_score) begin
winner <= 2'b01; // 黄队获胜
yellow_led <= 2'b11; // 黄队LED灯闪烁
end else if (yellow_score < red_score) begin
winner <= 2'b10; // 红队获胜
red_led <= 2'b11; // 红队LED灯闪烁
end else begin
winner <= 2'b00; // 平局
end
end
// 黄队键盘输入
case (keys[1:0])
2'b01: yellow_input <= 2'b01; // 黄队加1分
2'b10: yellow_input <= 2'b10; // 黄队加2分
2'b11: yellow_input <= 2'b11; // 黄队加3分
default: yellow_input <= 2'b00;
endcase
// 红队键盘输入
case (keys[3:2])
2'b01: red_input <= 2'b01; // 红队加1分
2'b10: red_input <= 2'b10; // 红队加2分
2'b11: red_input <= 2'b11; // 红队加3分
default: red_input <= 2'b00;
endcase
// 黄队分数更新
case (yellow_input)
2'b01: yellow_score <= yellow_score + 8'b00000001; // 加1分
2'b10: yellow_score <= yellow_score + 8'b00000010; // 加2分
2'b11: yellow_score <= yellow_score + 8'b00000011; // 加3分
default: yellow_score <= yellow_score;
endcase
// 红队分数更新
case (red_input)
2'b01: red_score <= red_score + 8'b00000001; // 加1分
2'b10: red_score <= red_score + 8'b00000010; // 加2分
2'b11: red_score <= red_score + 8'b00000011; // 加3分
default: red_score <= red_score;
endcase
// 黄队LED灯
case (yellow_input)
2'b01: yellow_led <= 2'b01; // 加1分
2'b10: yellow_led <= 2'b10; // 加2分
2'b11: yellow_led <= 2'b11; // 加3分
default: yellow_led <= 2'b00;
endcase
// 红队LED灯
case (red_input)
2'b01: red_led <= 2'b01; // 加1分
2'b10: red_led <= 2'b10; // 加2分
2'b11: red_led <= 2'b11; // 加3分
default: red_led <= 2'b00;
endcase
end
end
endmodule
```
此外,您还需要编写一个顶层模块,将FPGA的输入输出端口与这个模块连接起来,并将该模块下载到FPGA中。
阅读全文
相关推荐
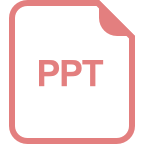



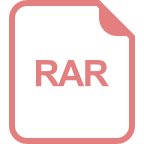
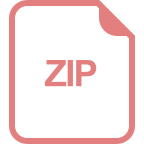
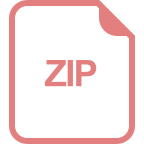
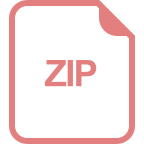
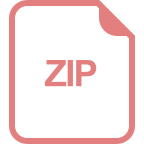
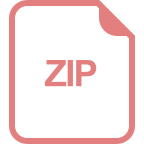
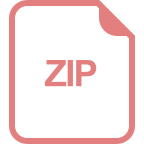
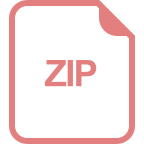