使用akshare获取股票数据强化学习获取最大收益完整代码
时间: 2024-05-07 14:22:06 浏览: 11
以下是一个简单的示例代码,使用 akshare 获取股票数据进行强化学习,以获取最大收益。
首先,我们需要安装 akshare 库。可以通过以下命令在命令行中安装:
```
pip install akshare
```
然后,我们需要准备股票数据。以下代码将获取某个股票的历史数据,并将其保存为 CSV 文件:
```python
import akshare as ak
import pandas as pd
stock_code = "sh600000"
start_date = "20100101"
end_date = "20210101"
stock_data = ak.stock_zh_a_hist(symbol=stock_code, start_date=start_date, end_date=end_date, adjust="qfq")
stock_data.to_csv(f"{stock_code}.csv", index=False)
```
接下来,我们将使用 Gym 库来创建一个强化学习环境。以下是一个简单的示例代码,用于创建一个股票交易环境:
```python
import gym
import numpy as np
import pandas as pd
class StockTradingEnv(gym.Env):
metadata = {'render.modes': ['human']}
def __init__(self, data, init_invest=20000):
super(StockTradingEnv, self).__init__()
self.data = data
self.init_invest = init_invest
self.current_step = None
self.reward_range = (0, self.init_invest)
self.action_space = gym.spaces.Discrete(3)
self.observation_space = gym.spaces.Box(low=0, high=1, shape=(6,))
def _next_observation(self):
obs = np.array([
self.data.loc[self.current_step, 'open'] / 1000,
self.data.loc[self.current_step, 'high'] / 1000,
self.data.loc[self.current_step, 'low'] / 1000,
self.data.loc[self.current_step, 'close'] / 1000,
self.data.loc[self.current_step, 'volume'] / 10000,
self.invested / self.init_invest
])
return obs
def _take_action(self, action):
current_price = np.random.uniform(
self.data.loc[self.current_step, 'low'],
self.data.loc[self.current_step, 'high']
)
sell_price = np.random.uniform(
self.data.loc[self.current_step, 'low'],
current_price
)
buy_price = np.random.uniform(
current_price,
self.data.loc[self.current_step, 'high']
)
if action == 0:
self.invested -= self.init_invest
self.balance += self.init_invest
elif action == 1:
self.invested += self.balance / current_price
self.balance = 0
elif action == 2:
self.balance += self.invested * sell_price
self.invested = 0
def step(self, action):
assert self.action_space.contains(action)
self._take_action(action)
self.current_step += 1
if self.current_step > len(self.data) - 1:
return self._next_observation(), 0, True, {}
reward = self.balance + self.invested * np.random.uniform(
self.data.loc[self.current_step, 'low'],
self.data.loc[self.current_step, 'high']
) - self.init_invest
done = self.balance + self.invested * self.data.loc[self.current_step, 'close'] <= 0
obs = self._next_observation()
return obs, reward, done, {}
def reset(self):
self.current_step = 0
self.balance = self.init_invest
self.invested = 0
return self._next_observation()
def render(self, mode='human', close=False):
profit = self.balance + self.invested * np.random.uniform(
self.data.loc[self.current_step, 'low'],
self.data.loc[self.current_step, 'high']
) - self.init_invest
print(f"Step: {self.current_step}")
print(f"Cash: {self.balance:.2f}")
print(f"Invested: {self.invested:.2f}")
print(f"Total: {self.balance + self.invested:.2f}")
print(f"Profit: {profit:.2f}")
```
最后,我们将使用 OpenAI Gym 和 TensorFlow 2.x 来训练一个神经网络,以学习交易策略。以下是一个简单的示例代码:
```python
import gym
import numpy as np
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout, LSTM
# Load data
data = pd.read_csv("sh600000.csv")
# Create environment
env = StockTradingEnv(data)
# Build model
model = Sequential()
model.add(LSTM(128, input_shape=(env.observation_space.shape[0],)))
model.add(Dropout(0.2))
model.add(Dense(64, activation='relu'))
model.add(Dense(32, activation='relu'))
model.add(Dense(env.action_space.n, activation='softmax'))
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Train model
epochs = 10
for epoch in range(epochs):
state = env.reset()
state = np.reshape(state, [1, env.observation_space.shape[0]])
for time in range(5000):
action_probs = model.predict(state)[0]
action = np.random.choice(env.action_space.n, p=action_probs)
next_state, reward, done, _ = env.step(action)
next_state = np.reshape(next_state, [1, env.observation_space.shape[0]])
if done:
target = reward
else:
target = reward + 0.95 * np.amax(model.predict(next_state)[0])
target_f = model.predict(state)
target_f[0][action] = target
model.fit(state, target_f, epochs=1, verbose=0)
state = next_state
if done:
print(f"Epoch: {epoch+1}/{epochs}, Profit: {reward:.2f}")
break
```
这个示例代码仅仅是一个简单的示例,实际上,你需要自己根据不同的需求来修改和完善它。
相关推荐
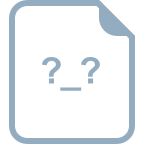
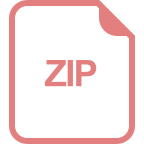














