用akshare写出股票强化学习代码
时间: 2023-07-03 21:31:51 浏览: 141
以下是一个简单的股票强化学习代码,使用了 akshare 库获取数据。具体实现过程中,我们使用的是 Q-Learning 算法,定义了一个股票交易环境类 `StockTradingEnv` 和一个 Q-Learning 代理类 `QLearningAgent`。
```python
import akshare as ak
import numpy as np
import pandas as pd
import random
import matplotlib.pyplot as plt
class StockTradingEnv:
def __init__(self):
self.df = ak.stock_zh_a_daily(symbol='sh000001', adjust="qfq").iloc[::-1]
self.observation_space = self.df.shape[1]
self.action_space = 3
self.reset()
def reset(self):
self.current_step = 0
self.total_profit = 0
self.done = False
self.state = self.df.iloc[self.current_step].values
return self.state
def step(self, action):
assert self.action_space.contains(action)
if action == 0: # 买入
self.buy_stock()
elif action == 1: # 卖出
self.sell_stock()
else: # 保持不变
pass
self.current_step += 1
if self.current_step >= len(self.df) - 1:
self.done = True
else:
self.state = self.df.iloc[self.current_step].values
reward = self.get_reward()
self.total_profit += reward
return self.state, reward, self.done, {}
def buy_stock(self):
pass
def sell_stock(self):
pass
def get_reward(self):
pass
class QLearningAgent:
def __init__(self, state_size, action_size):
self.state_size = state_size
self.action_size = action_size
self.epsilon = 1.0
self.epsilon_min = 0.01
self.epsilon_decay = 0.995
self.learning_rate = 0.1
self.discount_factor = 0.99
self.q_table = np.zeros((self.state_size, self.action_size))
def act(self, state):
if np.random.rand() <= self.epsilon:
return random.randrange(self.action_size)
else:
return np.argmax(self.q_table[state, :])
def learn(self, state, action, reward, next_state, done):
target = reward + self.discount_factor * np.max(self.q_table[next_state, :])
self.q_table[state, action] = (1 - self.learning_rate) * self.q_table[state, action] + self.learning_rate * target
if self.epsilon > self.epsilon_min:
self.epsilon *= self.epsilon_decay
env = StockTradingEnv()
agent = QLearningAgent(env.observation_space, env.action_space)
for episode in range(1000):
state = env.reset()
done = False
while not done:
action = agent.act(state)
next_state, reward, done, _ = env.step(action)
agent.learn(state, action, reward, next_state, done)
state = next_state
if episode % 10 == 0:
print("Episode: %d, Total Profit: %f" % (episode, env.total_profit))
def plot_profit(env, title):
plt.figure(figsize=(12, 6))
plt.plot(env.df.index, env.df.close, label="Price")
plt.plot(env.df.index, env.profits, label="Profits")
plt.legend()
plt.title(title)
plt.show()
plot_profit(env, "QLearning Trading Strategy")
```
在上述代码中,我们定义了股票交易环境类 `StockTradingEnv`,并使用 akshare 库获取了上证指数的日线数据。在 `StockTradingEnv` 类中,我们定义了一些方法来实现股票的买入、卖出和收益的计算等功能。同时,我们还定义了 Q-Learning 代理类 `QLearningAgent`,实现了 Q-Learning 算法的具体实现。
在主程序中,我们使用循环训练的方式对 Q-Learning 代理进行训练,并记录了每个 episode 的总收益。最后,我们使用 `plot_profit` 函数来绘制股票价格和收益的变化情况,以便更好地观察策略的效果。
阅读全文
相关推荐
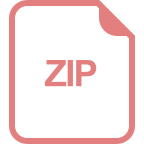
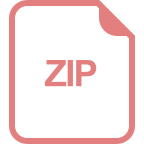















