def create_edge_adj(vertex_adj): ''' create an edge adjacency matrix from vertex adjacency matrix ''' vertex_adj.setdiag(0) edge_index = np.nonzero(sp.triu(vertex_adj, k=1)) num_edge = int(len(edge_index[0])) edge_name = [x for x in zip(edge_index[0], edge_index[1])] edge_adj = np.zeros((num_edge, num_edge)) for i in range(num_edge): for j in range(i, num_edge): if len(set(edge_name[i]) & set(edge_name[j])) == 0: edge_adj[i, j] = 0 else: edge_adj[i, j] = 1 adj = edge_adj + edge_adj.T np.fill_diagonal(adj, 1) return sp.csr_matrix(adj), edge_name
时间: 2024-04-18 22:23:48 浏览: 124
这个函数的作用是从给定的顶点邻接矩阵创建一个边邻接矩阵。顶点邻接矩阵用来表示图中顶点之间的连接关系,而边邻接矩阵则表示图中边之间的连接关系。函数通过对给定的顶点邻接矩阵进行处理,生成对应的边邻接矩阵。
首先,函数将顶点邻接矩阵的对角线元素设置为0,因为对角线上的元素表示顶点与自身的连接关系,在边邻接矩阵中不需要考虑这种情况。
然后,函数使用`np.nonzero`函数找到顶点邻接矩阵中非零元素所在的行列索引,这些索引表示图中存在的边。这些边的索引被存储在`edge_index`中。
接下来,函数计算边的数量,并将边的索引存储在`edge_name`列表中。`edge_name`列表中的每个元素是一个包含两个值的元组,表示一条边连接的两个顶点的索引。
然后,函数创建一个大小为`(num_edge, num_edge)`的全零边邻接矩阵。
接下来,函数使用两层循环来遍历边邻接矩阵中的每个元素。对于每对边,函数检查它们所连接的顶点是否有公共的顶点。如果没有公共顶点,则边邻接矩阵中对应位置的值为0;否则,值为1。
最后,函数将边邻接矩阵与其转置相加,得到对称的边邻接矩阵,并将对角线上的元素设置为1,表示每条边都与自身相连。
最后,函数返回一个使用稀疏矩阵表示的边邻接矩阵和边的名称列表。
相关问题
import heapqclass Graph: def __init__(self, vertices): self.vertices = vertices self.adjacency_list = {v: [] for v in vertices} def add_edge(self, u, v, weight): self.adjacency_list[u].append((v, weight)) self.adjacency_list[v].append((u, weight)) def dijkstra(self, source): heap = [(0, source)] visited = set() shortest_distances = {v: float('inf') for v in self.vertices} shortest_distances[source] = 0 while heap: (dist, current_vertex) = heapq.heappop(heap) if current_vertex in visited: continue visited.add(current_vertex) for (neighbor, weight) in self.adjacency_list[current_vertex]: distance = dist + weight if distance < shortest_distances[neighbor]: shortest_distances[neighbor] = distance heapq.heappush(heap, (distance, neighbor)) for v in self.vertices: print(f"{source}->{v}的最短路径为:{v} 长度:{shortest_distances[v]}")# 测试代码vertices = ['A', 'B', 'C', 'D', 'E']graph = Graph(vertices)graph.add_edge('A', 'B', 6)graph.add_edge('A', 'D', 1)graph.add_edge('B', 'C', 5)graph.add_edge('B', 'D', 2)graph.add_edge('B', 'E', 2)graph.add_edge('C', 'E', 5)graph.add_edge('D', 'E', 1)graph.dijkstra('A')
这是一个使用 Dijkstra 算法求解最短路径的代码。假设有一个无向图,使用字典 `adjacency_list` 存储每个顶点的邻接表。在 `dijkstra` 方法中,首先将起始顶点放入堆中,然后使用 `visited` 集合记录已经访问过的顶点。使用字典 `shortest_distances` 记录每个顶点到起始顶点的最短距离,初始值为正无穷。当从堆中弹出一个顶点时,如果该顶点已经被访问过,则直接跳过。否则遍历该顶点的所有邻居,计算它们到起始顶点的距离,并更新 `shortest_distances` 和堆。最后,打印出起始顶点到每个顶点的最短路径长度。
代码中给出了一个简单的测试例子,使用节点 A、B、C、D、E 构建一个无向图,并从起点 A 开始求解最短路径。
Function: Creat an undirected graph Args: *G: Pointer to a gragh *ver: An array of vertex value *edge: An array of edges Description: Construct a graph which is stored in the form of an adjacency list */ void creatGraph(Graph* G, VertexType ver[], VertexType edge[][2]) { }
好的,下面是 `creatGraph` 函数的代码实现:
```c++
void creatGraph(Graph* G, VertexType ver[], VertexType edge[][2]) {
int i, j, k;
ArcNode* p;
for (i = 0; i < MAX_VERTEX_NUM; i++) {
G->adjList[i].firstArc = NULL;
}
G->numVertexes = sizeof(ver) / sizeof(VertexType);
G->numEdges = sizeof(edge) / sizeof(VertexType) / 2;
for (i = 0; i < G->numVertexes; i++) {
G->adjList[i].data = ver[i];
}
for (k = 0; k < G->numEdges; k++) {
i = LocateVertex(G, edge[k][0]);
j = LocateVertex(G, edge[k][1]);
p = (ArcNode*)malloc(sizeof(ArcNode));
p->adjvex = j;
p->nextArc = G->adjList[i].firstArc;
G->adjList[i].firstArc = p;
p = (ArcNode*)malloc(sizeof(ArcNode));
p->adjvex = i;
p->nextArc = G->adjList[j].firstArc;
G->adjList[j].firstArc = p;
}
}
```
其中,`LocateVertex` 函数用于查找顶点在邻接表中的位置,代码如下:
```c++
int LocateVertex(Graph* G, VertexType v) {
int i;
for (i = 0; i < G->numVertexes; i++) {
if (G->adjList[i].data == v) {
return i;
}
}
return -1;
}
```
需要注意的是,在这个函数中,我们假设邻接表中的顶点值不会重复。
阅读全文
相关推荐
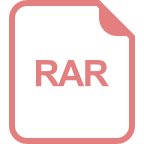
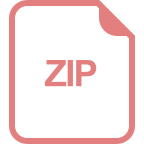
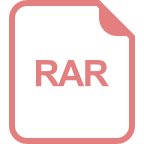
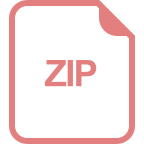
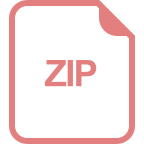
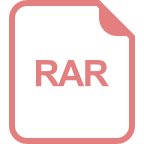










