下列代码是什么意思static Stu[] Stu = new Stu[8];//定义学生数组,根据题目要求容量为8 static int number = 0; static Scanner scanner = new Scanner(System.in); public static void main(String args[]) { int choice; while (true) { System.out.println("1 添加新的学生信息"); System.out.println("2 展示学生信息"); System.out.println("3 查询学生信息"); System.out.println("4 学生信息统计信息"); System.out.println("5 退出"); System.out.println("请输入输入相应数字选择要进行的操作:"); choice = scanner.nextInt(); if (choice == 5) break; switch (choice) { case 1: add(); break;//添加学生信息 case 2: show(); break;//展示学生信息 case 3: query(); break;//查询学生信息 case 4: tongji(); break;//统计籍贯为陕西的学生人数,以及男生和女生的人数; } } System.out.println("\n结束"); }
时间: 2023-02-11 20:42:34 浏览: 205
这段代码实现了一个学生信息管理系统的基本功能。
它定义了一个学生数组,容量为8,并且初始化了一个Scanner对象,以便在程序运行时获取用户的输入。
在 main 函数中, 使用一个 while 循环来提供多次操作的机会,每次循环会输出一个菜单,提供用户输入要执行的操作的数字。
根据用户输入的数字,使用switch语句分别调用不同的函数来实现不同的功能,包括添加学生信息、展示学生信息、查询学生信息、学生信息统计。
当用户输入5的时候,程序会break退出循环,输出结束信息。
相关问题
public static void main(String[] args) { //给出n名学生 Scanner sc=new Scanner(System.in); int n=sc.nextInt(); //开辟结构体数组 student stu[]=new student[n]; for (int i = 0; i < n; i++) { stu[i]=new student();//<<注意这里 stu[i].name=sc.next(); stu[i].score=sc.nextInt(); }
这段代码是用 Java 语言实现的,主要是用来读入 n 名学生的姓名和分数,然后将它们存储到一个名为 stu 的结构体数组中。具体来说,代码首先使用 Scanner 类读入一个整数 n,表示学生的数量。然后,它创建了一个长度为 n 的学生数组 stu,并使用 for 循环依次读入每一个学生的姓名和分数,存储到对应的结构体对象中。值得注意的是,在每一次循环中,代码都需要使用 new 操作符为 stu 数组中的每一个元素创建一个新的 student 对象,以便存储该学生的信息。
为什么以下代码的学生测试类显示灰色,请找出这段代码不能运行的原因,并修改package MianXiangDuiXiangDemo3; public class StudentsTest { public static boolean main(String[] args) { //创建数组 Students[] arr = new Students[3]; //创建学生对象 Students stu1 = new Students("财", 1, 24); Students stu2 = new Students("来", 2, 26); Students stu3 = new Students("旺", 3, 21); //添加到数组 arr[0] = stu1; arr[1] = stu2; arr[2] = stu3; //添加一个学生对象 Students stu4 = new Students("盛", 4, 23); //唯一性判断,已存在,则不添加,不存在,则加 boolean flag = contains(arr, stu4.getId()); if (flag) { //存在 System.out.println("id存在,请重新添加"); } else { //不存在 //数组存满,则创建一个新的数组,新的数组长度 = +1 //数组没满,直接添加 int count = getcount(arr); if (count == arr.length) { //数组存满 //创建一个新的数组,新的数组长度 = +1 Students[] newArr = creatNewArr(arr); newArr[count] = stu4; printArr(newArr); } else { //数组没满 arr[count] = stu4; printArr(arr); } } return flag; }
以下是修改后的代码:
```java
package MianXiangDuiXiangDemo3;
public class StudentsTest {
public static void main(String[] args) {
//创建数组
Students[] arr = new Students[3];
//创建学生对象
Students stu1 = new Students("财", 1, 24);
Students stu2 = new Students("来", 2, 26);
Students stu3 = new Students("旺", 3, 21);
//添加到数组
arr[0] = stu1;
arr[1] = stu2;
arr[2] = stu3;
//添加一个学生对象
Students stu4 = new Students("盛", 4, 23);
//唯一性判断,已存在,则不添加,不存在,则加
boolean flag = contains(arr, stu4.getId());
if (flag) { //存在
System.out.println("id存在,请重新添加");
} else { //不存在
//数组存满,则创建一个新的数组,新的数组长度 = +1
//数组没满,直接添加
int count = getCount(arr);
if (count == arr.length) { //数组存满
//创建一个新的数组,新的数组长度 = +1
Students[] newArr = createNewArr(arr);
newArr[count] = stu4;
printArr(newArr);
} else { //数组没满
arr[count] = stu4;
printArr(arr);
}
}
}
public static boolean contains(Students[] arr, int id) {
for (Students s : arr) {
if (s != null && s.getId() == id) {
return true;
}
}
return false;
}
public static int getCount(Students[] arr) {
int count = 0;
for (Students s : arr) {
if (s != null) {
count++;
}
}
return count;
}
public static Students[] createNewArr(Students[] arr) {
Students[] newArr = new Students[arr.length + 1];
for (int i = 0; i < arr.length; i++) {
newArr[i] = arr[i];
}
return newArr;
}
public static void printArr(Students[] arr) {
for (Students s : arr) {
if (s != null) {
System.out.println(s);
}
}
}
}
```
原因是原代码中的 `main()` 方法返回类型为 `boolean`,而不是 `void`,因此无法运行。同时,还需要将 `main()` 方法中的唯一性判断、计算数组大小、创建新数组和打印数组的代码移至其他方法中,并进行相应修改。
阅读全文
相关推荐
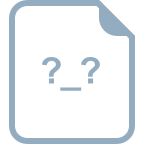
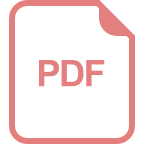
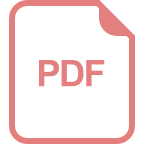













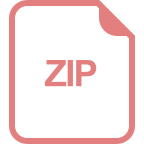