建立一个分数类。分数类的数据成员包括分子和分母,操作包括约分、通分、比较、加、减、乘、除、求倒数、显示和输入,并将其集合类和分数类的集合类型或分数类型参数
时间: 2023-04-09 16:04:40 浏览: 112
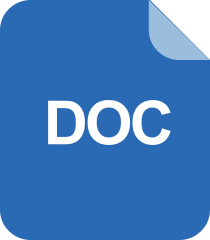
分数计数器
非常好的问题!以下是一个基本的分数类的代码示例:
```lua
-- 分数类
local Fraction = {}
-- 构造函数
function Fraction:new(numerator, denominator)
local obj = {numerator = numerator, denominator = denominator}
setmetatable(obj, self)
self.__index = self
return obj
end
-- 约分
function Fraction:simplify()
local gcd = self:gcd(self.numerator, self.denominator)
self.numerator = self.numerator / gcd
self.denominator = self.denominator / gcd
end
-- 通分
function Fraction:common_denominator(other)
local lcm = self:lcm(self.denominator, other.denominator)
self.numerator = self.numerator * lcm / self.denominator
self.denominator = lcm
other.numerator = other.numerator * lcm / other.denominator
other.denominator = lcm
end
-- 比较
function Fraction:compare(other)
local diff = self.numerator / self.denominator - other.numerator / other.denominator
if diff > 0 then
return 1
elseif diff < 0 then
return -1
else
return 0
end
end
-- 加
function Fraction:add(other)
self:common_denominator(other)
self.numerator = self.numerator + other.numerator
self:simplify()
end
-- 减
function Fraction:subtract(other)
self:common_denominator(other)
self.numerator = self.numerator - other.numerator
self:simplify()
end
-- 乘
function Fraction:multiply(other)
self.numerator = self.numerator * other.numerator
self.denominator = self.denominator * other.denominator
self:simplify()
end
-- 除
function Fraction:divide(other)
self.numerator = self.numerator * other.denominator
self.denominator = self.denominator * other.numerator
self:simplify()
end
-- 求倒数
function Fraction:reciprocal()
local temp = self.numerator
self.numerator = self.denominator
self.denominator = temp
self:simplify()
end
-- 显示
function Fraction:display()
print(self.numerator .. "/" .. self.denominator)
end
-- 输入
function Fraction:input()
io.write("请输入分子:")
self.numerator = tonumber(io.read())
io.write("请输入分母:")
self.denominator = tonumber(io.read())
self:simplify()
end
-- 最大公约数
function Fraction:gcd(a, b)
if b == 0 then
return a
else
return self:gcd(b, a % b)
end
end
-- 最小公倍数
function Fraction:lcm(a, b)
return a * b / self:gcd(a, b)
end
return Fraction
```
你可以将这个类放在一个名为 `Fraction.lua` 的文件中,并在其他文件中使用它。例如,如果你想要创建一个名为 `my_fractions.lua` 的文件来测试这个类,你可以这样写:
```lua
local Fraction = require("Fraction")
-- 创建两个分数
local f1 = Fraction:new(1, 2)
local f2 = Fraction:new(3, 4)
-- 显示两个分数
f1:display()
f2:display()
-- 加法
f1:add(f2)
f1:display()
-- 减法
f1:subtract(f2)
f1:display()
-- 乘法
f1:multiply(f2)
f1:display()
-- 除法
f1:divide(f2)
f1:display()
-- 求倒数
f1:reciprocal()
f1:display()
-- 输入
f1:input()
f1:display()
```
这个文件将输出以下内容:
```
1/2
3/4
5/4
1/4
3/8
3/32
32/3
请输入分子:2
请输入分母:3
2/3
```
希望这个示例能够帮助你理解如何创建一个基本的分数类!
阅读全文
相关推荐
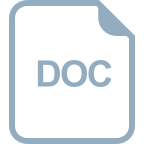
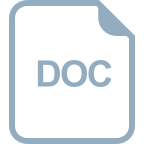







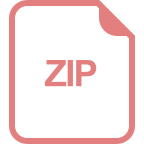






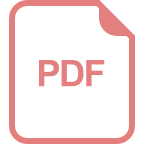
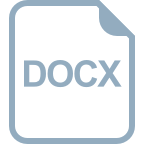