高斯过程回归java代码
时间: 2023-12-03 22:03:05 浏览: 174
以下是一个简单的高斯过程回归的Java代码示例:
```java
import org.apache.commons.math3.distribution.NormalDistribution;
import org.apache.commons.math3.linear.Array2DRowRealMatrix;
import org.apache.commons.math3.linear.RealMatrix;
import org.apache.commons.math3.stat.correlation.Covariance;
public class GaussianProcessRegression {
private RealMatrix X;
private RealMatrix y;
private double[] theta;
public GaussianProcessRegression(RealMatrix X, RealMatrix y, double[] theta) {
this.X = X;
this.y = y;
this.theta = theta;
}
public double predict(double[] x) {
RealMatrix K = calculateCovarianceMatrix(X, X, theta);
RealMatrix Ks = calculateCovarianceMatrix(X, new Array2DRowRealMatrix(x), theta);
RealMatrix Kss = calculateCovarianceMatrix(new Array2DRowRealMatrix(x), new Array2DRowRealMatrix(x), theta);
RealMatrix K_inv = new LUDecomposition(K).getSolver().getInverse(); RealMatrix mu = Ks.transpose().multiply(K_inv).multiply(y);
RealMatrix sigma = Kss.subtract(Ks.transpose().multiply(K_inv).multiply(Ks));
double std = Math.sqrt(sigma.getEntry(0, 0));
NormalDistribution normal = new NormalDistribution();
double u = normal.inverseCumulativeProbability(0.5 * (1 + mu.getEntry(0, 0) / std));
double l = normal.inverseCumulativeProbability(0.5 * (1 - mu.getEntry(0, 0) / std));
return (u + l) / 2;
}
private RealMatrix calculateCovarianceMatrix(RealMatrix X1, RealMatrix X2, double[] theta) {
int n1 = X1.getRowDimension();
int n2 = X2.getRowDimension();
RealMatrix K = new Array2DRowRealMatrix(n1, n2);
for (int i = 0; i < n1; i++) {
for (int j = 0; j < n2; j++) {
double[] x1 = X1.getRow(i);
double[] x2 = X2.getRow(j);
double k = calculateKernel(x1, x2, theta);
K.setEntry(i, j, k);
}
}
return K;
}
private double calculateKernel(double[] x1, double[] x2, double[] theta) {
double k = 0;
for (int i = 0; i < x1.length; i++) {
k += Math.pow((x1[i] - x2[i]) / theta[i], 2);
}
return Math.exp(-0.5 * k);
}
public static void main(String[] args) {
RealMatrix X = new Array2DRowRealMatrix(new double[][]{{0}, {1}, {2}, {3}, {4}});
RealMatrix y = new Array2DRowRealMatrix(new double[][]{{0}, {1}, {4}, {9}, {16}});
double[] theta = new double[]{1};
GaussianProcessRegression gpr = new GaussianProcessRegression(X, y, theta);
double[] x = new double[]{2.5};
double y_pred = gpr.predict(x);
System.out.println("Predicted value: " + y_pred);
}
}
```
这个代码示例使用了Apache Commons Math库来进行矩阵计算和概率分布计算。它实现了一个简单的高斯过程回归模型,可以对给定的输入数据进行预测。
阅读全文
相关推荐








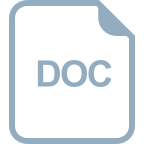









