请帮我用python语言写一个统计csv文件中每一行中文字的个数,并按照中文字数的个数作比较,并用pyecharts表的方式将比较的结果由大到小呈现出来
时间: 2024-02-17 14:05:11 浏览: 151
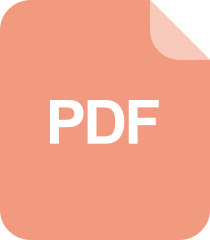
对Python 多线程统计所有csv文件的行数方法详解
好的,可以使用Python的pandas和pyecharts库来完成这个任务。
首先需要安装pandas和pyecharts库,可以使用以下命令进行安装:
```python
pip install pandas pyecharts
```
然后,我们可以使用pandas库读取csv文件中的数据,并计算每一行中中文字符的个数:
```python
import pandas as pd
import re
# 读取csv文件
df = pd.read_csv('data.csv')
# 计算每一行中中文字符的个数
def count_chinese_chars(row):
chinese_pattern = re.compile(u'[\u4e00-\u9fa5]')
chinese_chars = chinese_pattern.findall(row)
return len(chinese_chars)
df['chinese_chars_count'] = df['text'].apply(count_chinese_chars)
```
接下来,我们可以使用pandas库的groupby方法按照中文字数的个数进行分组:
```python
# 按照中文字数的个数进行分组
grouped = df.groupby('chinese_chars_count').count()
```
最后,我们可以使用pyecharts库将比较的结果由大到小呈现出来:
```python
from pyecharts import Bar
# 将比较的结果由大到小呈现出来
bar = Bar("每行中中文字符的个数统计")
bar.add("", grouped.index, grouped['text'], is_stack=True, is_label_show=True)
bar.render("chinese_chars_count.html")
```
完整代码如下:
```python
import pandas as pd
import re
from pyecharts import Bar
# 读取csv文件
df = pd.read_csv('data.csv')
# 计算每一行中中文字符的个数
def count_chinese_chars(row):
chinese_pattern = re.compile(u'[\u4e00-\u9fa5]')
chinese_chars = chinese_pattern.findall(row)
return len(chinese_chars)
df['chinese_chars_count'] = df['text'].apply(count_chinese_chars)
# 按照中文字数的个数进行分组
grouped = df.groupby('chinese_chars_count').count()
# 将比较的结果由大到小呈现出来
bar = Bar("每行中中文字符的个数统计")
bar.add("", grouped.index, grouped['text'], is_stack=True, is_label_show=True)
bar.render("chinese_chars_count.html")
```
注意:需要将data.csv改为实际的csv文件名。生成的图表会保存为chinese_chars_count.html文件,可以使用浏览器打开查看。
阅读全文
相关推荐
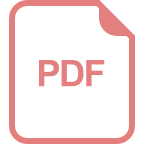
















