db.getCollection("website_sort").aggregate([ { $match: { "websiteCategoryId": "63c27b11d5957b16c46dc17e" } }, { "$project": { id: { "$toObjectId": "$websiteListId" } } }, { "$lookup": { "from": "website_list_cs", "localField": "id", //A表需要关联的键 "foreignField": "_id", //B表需要关联的键 "as": "B_list" //B表的别名,下面输出B表字段时用到 } }, { $project: { id: 1, "B_list.title": 1, "B_list.desc": 1, "B_list.labels": 1, "B_list.link": 1, "B_list.logo": 1, "B_list.vpn": 1, "B_list.snapshot": 1, "B_list.recommend": 1, "B_list.vpn": 1, "B_list.status": 1 } }, { "$sort": { "id": 1 } } ]);转java
时间: 2023-03-04 20:12:51 浏览: 74
Here's an example Java code to execute the given MongoDB aggregation query:
```
import com.mongodb.client.AggregateIterable;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.Filters;
import org.bson.Document;
import java.util.Arrays;
public class MongoAggregationExample {
public static void main(String[] args) {
// Create MongoDB client
MongoClient mongoClient = MongoClients.create("mongodb://localhost:27017");
// Get reference to the database
MongoDatabase database = mongoClient.getDatabase("yourDatabaseName");
// Build the aggregation pipeline
AggregateIterable<Document> result = database.getCollection("website_sort").aggregate(Arrays.asList(
new Document("$match", Filters.eq("websiteCategoryId", "63c27b11d5957b16c46dc17e")),
new Document("$project", new Document("id", new Document("$toObjectId", "$websiteListId"))),
new Document("$lookup", new Document("from", "website_list_cs")
.append("localField", "id")
.append("foreignField", "_id")
.append("as", "B_list")),
new Document("$project", new Document("id", 1)
.append("B_list.title", 1)
.append("B_list.desc", 1)
.append("B_list.labels", 1)
.append("B_list.link", 1)
.append("B_list.logo", 1)
.append("B_list.vpn", 1)
.append("B_list.snapshot", 1)
.append("B_list.recommend", 1)
.append("B_list.vpn", 1)
.append("B_list.status", 1)),
new Document("$sort", new Document("id", 1))
));
// Print the result
for (Document doc : result) {
System.out.println(doc.toJson());
}
// Close the MongoDB client
mongoClient.close();
}
}
```
Make sure to replace "yourDatabaseName" with the name of your actual database. Also, don't forget to add the necessary MongoDB Java driver to your project's dependencies.
相关推荐
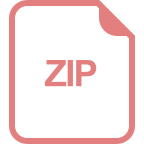
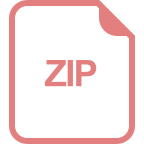
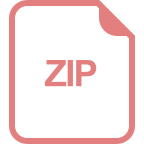














