用 python写一个线程池
时间: 2023-10-24 13:13:46 浏览: 36
以下是一个简单的线程池实现:
```python
import threading
from queue import Queue
class ThreadPool:
def __init__(self, num_threads):
self.num_threads = num_threads
self.task_queue = Queue()
self.threads = []
def start(self):
for i in range(self.num_threads):
thread = threading.Thread(target=self._worker)
thread.start()
self.threads.append(thread)
def submit(self, func, *args, **kwargs):
self.task_queue.put((func, args, kwargs))
def _worker(self):
while True:
task = self.task_queue.get()
if task is None:
break
func, args, kwargs = task
try:
func(*args, **kwargs)
except Exception as e:
print(e)
def join(self):
for i in range(self.num_threads):
self.task_queue.put(None)
for thread in self.threads:
thread.join()
```
使用示例:
```python
def task(name):
print('Task {} started'.format(name))
time.sleep(1)
print('Task {} finished'.format(name))
pool = ThreadPool(2)
pool.start()
pool.submit(task, 'A')
pool.submit(task, 'B')
pool.submit(task, 'C')
pool.join()
```
输出:
```
Task A started
Task B started
Task A finished
Task C started
Task B finished
Task C finished
```
相关推荐
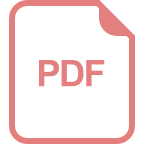
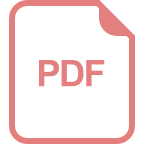
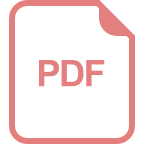














