java7.定义一个车(vehiele)基类,有Run、Stop等成员函数,由此派生出自行车(bicycle)类、汽车(motorcar)类。它们都有自己的Run、Stop等成员函数。
时间: 2023-06-09 09:04:03 浏览: 126
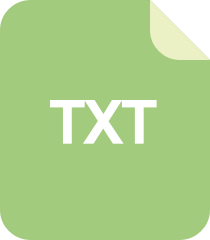
用c++ 定义一个车(Vehicle)基类,有Run,Stop等成员函数,由此派生出自行车(bicycle)类,汽车(motorcar)
好的,根据您的要求,以下是Java代码实现:
public class Vehicle {
public void run() {
System.out.println("Vehicle is running.");
}
public void stop() {
System.out.println("Vehicle has stopped.");
}
}
public class Bicycle extends Vehicle {
@Override
public void run() {
System.out.println("Bicycle is pedaling.");
}
@Override
public void stop() {
System.out.println("Bicycle has come to a stop.");
}
}
public class Motorcar extends Vehicle {
@Override
public void run() {
System.out.println("Motorcar is driving.");
}
@Override
public void stop() {
System.out.println("Motorcar has come to a stop.");
}
}
请注意,如果您需要其他变量或方法,请告诉我,我会尽力满足您的要求。
阅读全文
相关推荐
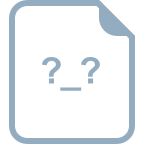
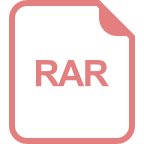












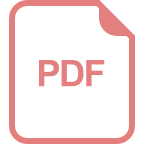
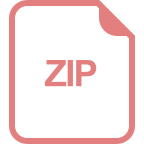