写一个ViT的完整代码
时间: 2023-03-20 08:02:36 浏览: 87
以下是一个基于PyTorch实现的ViT模型的完整代码:
```
import torch
import torch.nn as nn
from einops.layers.torch import Rearrange
class MLP(nn.Module):
def __init__(self, in_features, hidden_features, out_features, dropout_prob):
super().__init__()
self.fc1 = nn.Linear(in_features, hidden_features)
self.fc2 = nn.Linear(hidden_features, out_features)
self.dropout = nn.Dropout(dropout_prob)
self.gelu = nn.GELU()
def forward(self, x):
x = self.fc1(x)
x = self.gelu(x)
x = self.dropout(x)
x = self.fc2(x)
x = self.dropout(x)
return x
class ViT(nn.Module):
def __init__(self, image_size, patch_size, num_classes, dim, depth, heads, mlp_dim, dropout_prob):
super().__init__()
assert image_size % patch_size == 0, "Image dimensions must be divisible by the patch size."
num_patches = (image_size // patch_size) ** 2
patch_dim = 3 * patch_size ** 2
self.patch_size = patch_size
self.embedding = nn.Linear(patch_dim, dim)
self.cls_token = nn.Parameter(torch.randn(1, 1, dim))
self.positional_encoding = nn.Parameter(torch.randn(1, num_patches + 1, dim))
self.dropout = nn.Dropout(dropout_prob)
self.transformer = nn.ModuleList([
nn.TransformerEncoderLayer(d_model=dim, nhead=heads, dim_feedforward=mlp_dim, dropout=dropout_prob)
for _ in range(depth)
])
self.layer_norm = nn.LayerNorm(dim)
self.fc = nn.Linear(dim, num_classes)
def forward(self, x):
x = Rearrange('b c (h p1) (w p2) -> b (h w) (p1 p2 c)', p1=self.patch_size, p2=self.patch_size)(x)
x = self.embedding(x)
cls_token = self.cls_token.expand(x.shape[0], -1, -1)
x = torch.cat((cls_token, x), dim=1)
x = x + self.positional_encoding
x = self.dropout(x)
for transformer_layer in self.transformer:
x = transformer_layer(x)
x = x[:, 0]
x = self.layer_norm(x)
x = self.fc(x)
return x
```
该模型包含一个ViT类和一个MLP类,其中ViT类是主要的模型类,MLP类是ViT中所使用的多层感知机。在ViT类中,输入图像被首先被切成大小为patch_size x patch_size的小块,然后通过线性层进行嵌入。之后,一个位置编码被加到嵌入后的向量上,位置编码是一个可学习的参数。接下来,这些向量经过若干个Transformer Encoder层的处理。在Transformer Encoder层的输出中,第一个位置的向量被视为类别向量,最后经过一些标准的全局平均池化和线性变换后,最终输出分类结果。
相关推荐
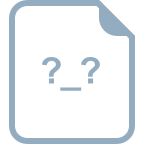














