写一段VIT模型的pytorch代码
时间: 2023-12-15 19:53:37 浏览: 32
好的,以下是使用PyTorch实现Vision Transformer(VIT)模型的示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class ViT(nn.Module):
def __init__(self, img_size, patch_size, emb_size, num_heads, num_layers, num_classes):
super(ViT, self).__init__()
self.patch_size = patch_size
self.num_patches = (img_size // patch_size) ** 2
self.proj = nn.Conv2d(3, emb_size, kernel_size=patch_size, stride=patch_size)
self.cls_token = nn.Parameter(torch.randn(1, 1, emb_size))
self.pos_emb = nn.Parameter(torch.randn(1, self.num_patches + 1, emb_size))
self.blocks = nn.ModuleList([TransformerBlock(emb_size, num_heads) for _ in range(num_layers)])
self.mlp_head = nn.Sequential(
nn.LayerNorm(emb_size),
nn.Linear(emb_size, num_classes)
)
def forward(self, x):
x = self.proj(x)
x = x.flatten(2).transpose(1, 2)
cls_tokens = self.cls_token.expand(x.shape[0], -1, -1)
x = torch.cat((cls_tokens, x), dim=1)
x += self.pos_emb[:, :(self.num_patches + 1)]
for block in self.blocks:
x = block(x)
x = x.mean(dim=1)
x = self.mlp_head(x)
return x
class TransformerBlock(nn.Module):
def __init__(self, emb_size, num_heads, mlp_ratio=4.0):
super(TransformerBlock, self).__init__()
self.attention = nn.MultiheadAttention(emb_size, num_heads)
self.mlp = nn.Sequential(
nn.Linear(emb_size, int(emb_size * mlp_ratio)),
nn.GELU(),
nn.Linear(int(emb_size * mlp_ratio), emb_size),
nn.Dropout(0.1)
)
self.norm1 = nn.LayerNorm(emb_size)
self.norm2 = nn.LayerNorm(emb_size)
def forward(self, x):
residual = x
x = self.norm1(x)
x = x.permute(1, 0, 2)
x, _ = self.attention(x, x, x)
x = x.permute(1, 0, 2)
x += residual
residual = x
x = self.norm2(x)
x = self.mlp(x)
x += residual
return x
```
相关推荐
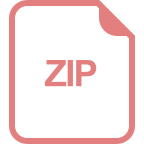
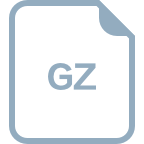
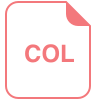
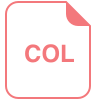












