# 定义二叉搜索树节点结构体class TreeNode: def __init__(self, val): self.val = val self.left = None self.right = None self.parent = None# 按输入顺序建立二叉搜索树def build_bst(arr): root = None for val in arr: root = insert(root, val) return root# 插入节点到二叉搜索树中def insert(root, val): if not root: return TreeNode(val) if val < root.val: root.left = insert(root.left, val) root.left.parent = root else: root.right = insert(root.right, val) root.right.parent = root return root# 搜索节点并输出其父节点的值def search(root, x): if not root: return "It does not exist." if root.val == x: if not root.parent: return "It doesn't have parent." else: return root.parent.val elif root.val > x: return search(root.left, x) else: return search(root.right, x)# 读取输入n = int(input())arr = list(map(int, input().split()))x = int(input())# 建立二叉搜索树root = build_bst(arr)# 搜索节点并输出其父节点的值print(search(root, x))显示该代码Traceback·(most·recent·call·last): ··File·"/tmp/a.py",·line·37,·in·<module> ····root·=·build_bst(arr) NameError:·name·'build_bst'·is·not·defined怎么解决
时间: 2023-12-02 13:03:22 浏览: 89
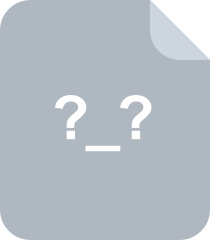
定义二叉搜索树类,封装查找、插入、删除操作

这个错误提示意味着在当前的作用域中找不到名为 `build_bst` 的函数。这可能是因为你没有将这个函数定义在与主程序同一作用域中。
你可以将 `build_bst` 函数定义在与主程序同一作用域中,或者将其定义在一个单独的文件中,并在主程序中引入它。例如,你可以将 `build_bst` 函数保存在一个名为 `bst.py` 的文件中,然后在主程序中使用以下代码来引入它:
```python
from bst import build_bst
# 读取输入
n = int(input())
arr = list(map(int, input().split()))
x = int(input())
# 建立二叉搜索树
root = build_bst(arr)
# 搜索节点并输出其父节点的值
print(search(root, x))
```
请确保 `bst.py` 文件与主程序文件在同一目录下,并且函数名和参数与主程序中的调用匹配。
阅读全文
相关推荐
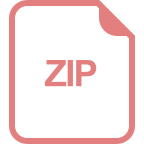
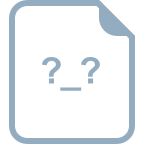
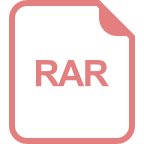
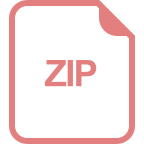
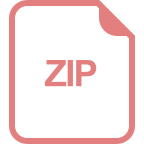
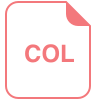
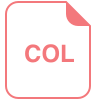










