python使用数据为影像数据5个影像分为短视频5个,对视频信息进行自动分析。影像里出现人脸的开始时间和结束时间记录到log文件里,名称为face.log
时间: 2024-03-26 12:42:05 浏览: 21
这个问题可以使用Python语言进行解决。下面是一种实现方案:
1. 安装必要的Python库:我们需要安装OpenCV和face_recognition这两个库。可以使用pip命令进行安装:
```python
pip install opencv-python
pip install face_recognition
```
2. 加载数据:将5个影像数据读入程序中,可以使用OpenCV中的VideoCapture类进行读取。
```python
import cv2
# 读取视频文件
video_capture = cv2.VideoCapture('video.mp4')
# 读取视频的每一帧
fps = video_capture.get(cv2.CAP_PROP_FPS)
frame_count = int(video_capture.get(cv2.CAP_PROP_FRAME_COUNT))
for i in range(frame_count):
ret, frame = video_capture.read()
if not ret:
break
# 处理每一帧,进行人脸识别
...
```
3. 进行人脸识别:使用face_recognition库进行人脸识别,检测每一帧中的人脸,并记录人脸出现的开始时间和结束时间。
```python
import face_recognition
import time
# 识别每一帧中的人脸
face_locations = face_recognition.face_locations(frame, model="cnn")
# 记录人脸出现的时间
if len(face_locations) > 0:
if not face_detected:
start_time = time.time()
face_detected = True
else:
if face_detected:
end_time = time.time()
face_detected = False
# 将人脸出现的开始时间和结束时间记录到face.log文件中
with open('face.log', 'a') as f:
f.write('face detected from {:.2f}s to {:.2f}s\n'.format(start_time, end_time))
```
4. 完整代码:
```python
import cv2
import face_recognition
import time
# 读取视频文件
video_capture = cv2.VideoCapture('video.mp4')
# 读取视频的每一帧
fps = video_capture.get(cv2.CAP_PROP_FPS)
frame_count = int(video_capture.get(cv2.CAP_PROP_FRAME_COUNT))
face_detected = False
for i in range(frame_count):
ret, frame = video_capture.read()
if not ret:
break
# 识别每一帧中的人脸
face_locations = face_recognition.face_locations(frame, model="cnn")
# 记录人脸出现的时间
if len(face_locations) > 0:
if not face_detected:
start_time = time.time()
face_detected = True
else:
if face_detected:
end_time = time.time()
face_detected = False
# 将人脸出现的开始时间和结束时间记录到face.log文件中
with open('face.log', 'a') as f:
f.write('face detected from {:.2f}s to {:.2f}s\n'.format(start_time, end_time))
# 释放视频文件
video_capture.release()
```
这个程序会对指定的视频文件进行人脸识别,并将结果记录到face.log文件中。需要注意的是,这个程序只能识别存在于视频中的人脸,对于视频中不存在人脸的时间段,不会记录任何信息。
相关推荐
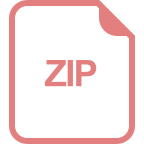
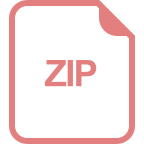
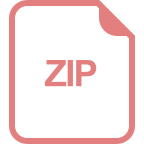














