单链表的创建,插入和删除,使用struct student和struct student *next的方法
时间: 2024-11-06 16:36:24 浏览: 20
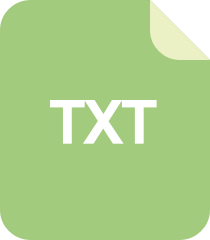
struct student
单链表是一种线性数据结构,它由一系列节点组成,每个节点包含两个部分:存储数据的域(如`struct student`)以及指向下一个节点的指针(`struct student *next`)。以下是使用`struct student`和`struct student *next`进行单链表操作的基本步骤:
1. 创建(构造)链表:
```c
// 定义结构体student
struct student {
char name[50];
int age;
struct student *next; // 指向下一个学生节点的指针
};
// 创建第一个节点(头节点)
struct student *head = (struct student*)malloc(sizeof(struct student));
head->name = "张三";
head->age = 20;
head->next = NULL; // 初始化头节点的next为NULL,表示链表为空
```
2. 插入节点:
- 在链表头部插入:假设我们要在头的位置插入新节点,可以这样做:
```c
struct student *newNode = (struct student*)malloc(sizeof(struct student));
newNode->name = "李四";
newNode->age = 22;
newNode->next = head; // 新节点的next指向当前头节点
head = newNode; // 更新头节点为新插入的新节点
```
- 在指定位置插入(例如插入到索引为i的位置)需要遍历找到那个位置再插入。
3. 删除节点:
- 删除某个学生的节点:
```c
struct student *temp = head;
while (temp != NULL && strcmp(temp->name, "要删除的学生名字") != 0) {
temp = temp->next;
}
if (temp != NULL) {
struct student *prev = temp->prev; // 获取前一个节点指针
if (prev != NULL) {
prev->next = temp->next; // 原前一个节点连接原后一个节点
} else { // 如果删除的是头节点
head = temp->next;
}
free(temp); // 释放已删除节点的内存
}
```
阅读全文
相关推荐
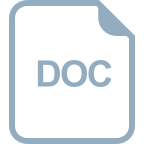
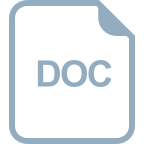















