z = model.predict(np.c_[xx.ravel(), yy.ravel()]) z = z.reshape(xx.shape) cs = plt.contourf(xx, yy, z)
时间: 2024-04-11 18:29:25 浏览: 263
这段代码是用来对模型进行预测并绘制等高线图的。首先,`model.predict()`函数用来对输入数据进行预测,其中`xx.ravel()`和`yy.ravel()`用来将二维数组`xx`和`yy`转换为一维数组。然后,通过`np.c_[]`函数将两个一维数组合并成一个二维数组作为模型的输入。预测结果`z`是一个一维数组,通过`z.reshape(xx.shape)`将其转换为与输入数据的维度相同的二维数组。最后,使用`plt.contourf()`函数绘制等高线图,其中`xx`和`yy`为网格数据,`z`为预测结果。
相关问题
import numpy as np import matplotlib.pyplot as plt from sklearn import datasets from sklearn import tree # 生成所有测试样本点 def make_meshgrid(x, y, h=.02): x_min, x_max = x.min() - 1, x.max() + 1 y_min, y_max = y.min() - 1, y.max() + 1 xx, yy = np.meshgrid(np.arange(x_min, x_max, h), np.arange(y_min, y_max, h)) return xx, yy # 对测试样本进行预测,并显示 def plot_test_results(ax, clf, xx, yy, **params): Z = clf.predict(np.c_[xx.ravel(), yy.ravel()]) Z = Z.reshape(xx.shape) ax.contourf(xx, yy, Z, **params) # 载入iris数据集(只使用前面连个特征) iris = datasets.load_iris() X_train,X_test,y_train,y_test = train_test_split(iris.data,iris.target,test_size = 0.20,random_state = 20) # 创建并训练决策树 clf = tree.DecisionTreeClassifier() # 决策树分类器 clf = clf.fit(X_train,y_train) # 生成所有测试样本点 plt.figure(dpi=200) # feature_names=iris.feature_names设置决策树中显示的特征名称 tree.plot_tree(clf,feature_names=iris.feature_names,class_names=iris.target_names) # 显示测试样本的分类结果 title = ('DecisionTreeClassifier') fig, ax = plt.subplots(figsize = (5, 5)) plt.subplots_adjust(wspace=0.4, hspace=0.4) plot_test_results(ax, clf, xx, yy, cmap=plt.cm.coolwarm, alpha=0.8) # 显示训练样本 ax.scatter(X0, X1, c=y, cmap=plt.cm.coolwarm, s=20, edgecolors='k') ax.set_xlim(xx.min(), xx.max()) ax.set_ylim(yy.min(), yy.max()) ax.set_xlabel('x1') ax.set_ylabel('x2') ax.set_xticks(()) ax.set_yticks(()) ax.set_title(title) plt.show()
这是一个使用决策树分类器对鸢尾花数据集进行训练和预测的Python代码。具体实现过程如下:
1.首先通过sklearn库中的datasets模块加载鸢尾花数据集,并使用train_test_split函数将数据集划分为训练集和测试集。
2.创建一个决策树分类器,并将其与训练集进行拟合。
3.使用plot_tree函数可视化生成的决策树,其中feature_names参数设置特征名称,class_names参数表示分类标签的名称。
4.使用make_meshgrid函数生成测试样本点的网格,并使用plot_test_results函数对测试样本进行预测,并将分类结果可视化显示在网格上。
5.最后使用scatter函数将训练样本显示在图像上,并设置相关的标题、坐标轴和刻度等属性。
该代码可以帮助我们更好地理解决策树分类器的工作原理,并对其在实际应用中的表现有更深入的了解。
def create_dataset(X, y, time_steps=1): Xs, ys = [], [] for i in range(len(X) - time_steps): Xs.append(X.iloc[i:(i + time_steps)].values) ys.append(y.iloc[i + time_steps]) return np.array(Xs), np.array(ys) TIME_STEPS = 10 X_train, y_train = create_dataset(train[['本车速度', '车头间距', '原车道前车速度']], train['本车加速度'], time_steps=TIME_STEPS) X_test, y_test = create_dataset(test[['本车速度', '车头间距', '原车道前车速度']], test['本车加速度'], time_steps=TIME_STEPS) train_predict = model.predict(X_train) test_predict = model.predict(X_test) train_predict = scaler.inverse_transform(train_predict) y_train = scaler.inverse_transform([y_train]) test_predict = scaler.inverse_transform(test_predict) y_test = scaler.inverse_transform([y_test])报错Traceback (most recent call last): File "C:\Users\马斌\Desktop\cnn测试\cnn改.py", line 49, in <module> train_predict = scaler.inverse_transform(train_predict) File "D:\python\python3.9.5\pythonProject\venv\lib\site-packages\sklearn\preprocessing\_data.py", line 541, in inverse_transform X -= self.min_ ValueError: non-broadcastable output operand with shape (110,1) doesn't match the broadcast shape (110,4)
这个错误通常会在使用sklearn的MinMaxScaler时出现。它表示输出操作数的形状不可广播到给定的形状。
根据你提供的代码,这个错误可能是由于y_train被转换为形状为(1, n_samples)的数组所致。你可以尝试使用ravel()函数将y_train的形状转换为(1, n_samples)的数组。
例如,将以下代码:
```
y_train = scaler.inverse_transform([y_train])
```
替换为:
```
y_train = scaler.inverse_transform(y_train.reshape(-1, 1)).ravel()
```
这应该可以解决这个问题。
如果你需要更具体的解决方案,请提供更多的上下文和代码。
阅读全文
相关推荐
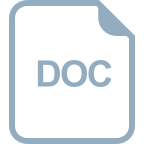
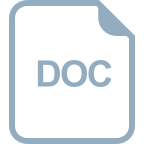
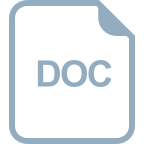













