2 个长度 n (n<=100000)数组 a,b; 所有的 ai + bj 从小到大排序后输出第 l 到第 r 项 输入第一行 n,l,r 第二行 n 个整数是 a 数组 。第三行 n 个整数是 b 数组 。 输出排序后第 l 到第 r 个数 完整的c++代码
时间: 2024-11-15 20:23:13 浏览: 1
为了实现这个功能,我们可以使用优先队列(通常用`std::priority_queue`或`std::vector`和`std::sort`配合)来存储计算结果并保证它们按照升序排列。这里是一个简单的C++代码示例:
```cpp
#include <iostream>
#include <vector>
#include <queue>
#include <algorithm>
// 假设我们定义一个辅助结构PairWithIndex用于存储计算结果和对应的索引
struct PairWithIndex {
int sum;
int index;
bool operator<(const PairWithIndex& other) const { return sum < other.sum; }
};
int main() {
int n, l, r;
std::cin >> n >> l >> r;
// 读取数组a和b
std::vector<int> a(n), b(n);
for (int i = 0; i < n; ++i) {
std::cin >> a[i];
}
for (int i = 0; i < n; ++i) {
std::cin >> b[i];
}
// 计算所有可能的和并将它们存入优先队列
std::priority_queue<PairWithIndex> pq;
for (int i = 0; i < n; ++i) {
pq.push({a[i] + b[i], i});
}
// 提取并打印第l到r个最小的和
std::vector<PairWithIndex> sorted_results(l - 1);
for (int i = 0; i < l - 1 && !pq.empty(); ++i) {
sorted_results[i] = pq.top();
pq.pop();
}
while (!pq.empty() && r > 0) {
if (pq.top().index != sorted_results[r - 1].index) {
sorted_results[r - 1] = pq.top();
--r;
} else {
pq.pop();
}
}
// 输出结果
for (const auto& result : sorted_results) {
std::cout << result.sum << " ";
}
std::cout << std::endl;
return 0;
}
```
在这个代码中,我们首先创建了一个优先队列`pq`来保存所有的和,并使用`operator<`确保它们按升序排列。然后我们从队列中取出前`l - 1`个元素放入结果向量`sorted_results`。接着,我们检查当前队列中的元素是否小于我们需要的第`r`个元素,如果是,我们就把该元素添加到结果中并减少`r`。当`r`变为0时,队列中剩余的元素都是大于等于所需的,所以无需再处理。
阅读全文
相关推荐
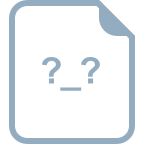
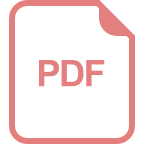
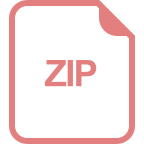
















