PSO优化SVRpython实现
时间: 2023-09-03 21:12:14 浏览: 165
以下是使用PSO优化SVR的Python实现示例:
导入所需的库:
```python
import numpy as np
from sklearn.svm import SVR
from pyswarm import pso
```
定义目标函数,即SVR的平均绝对误差(MAE):
```python
def svr_mae(x, X, y):
C = x[0]
gamma = x[1]
epsilon = x[2]
svr = SVR(C=C, gamma=gamma, epsilon=epsilon)
svr.fit(X, y)
y_pred = svr.predict(X)
mae = np.mean(np.abs(y - y_pred))
return mae
```
其中,x为优化变量,即SVR的超参数C、gamma和epsilon;X为特征矩阵,y为目标变量向量。
定义优化问题的边界:
```python
lb = [1, 0.0001, 0.0001] # lower bounds of C, gamma, and epsilon
ub = [100, 100, 1] # upper bounds of C, gamma, and epsilon
```
其中,lb和ub分别为超参数C、gamma和epsilon的下界和上界。
使用PSO求解最优超参数:
```python
xopt, fopt = pso(svr_mae, lb, ub, args=(X, y), swarmsize=100, maxiter=100, omega=0.5, phip=0.5, phig=0.5)
```
其中,swarmsize为粒子群大小,maxiter为最大迭代次数,omega、phip和phig为惯性权重、个体和全局学习因子。
最终得到的xopt就是最优超参数,fopt为目标函数的最小值(即MAE的最小值)。
完整代码示例:
```python
import numpy as np
from sklearn.svm import SVR
from pyswarm import pso
# define objective function
def svr_mae(x, X, y):
C = x[0]
gamma = x[1]
epsilon = x[2]
svr = SVR(C=C, gamma=gamma, epsilon=epsilon)
svr.fit(X, y)
y_pred = svr.predict(X)
mae = np.mean(np.abs(y - y_pred))
return mae
# define problem bounds
lb = [1, 0.0001, 0.0001] # lower bounds of C, gamma, and epsilon
ub = [100, 100, 1] # upper bounds of C, gamma, and epsilon
# generate random data
X = np.random.rand(100, 10)
y = np.random.rand(100)
# optimize SVR hyperparameters using PSO
xopt, fopt = pso(svr_mae, lb, ub, args=(X, y), swarmsize=100, maxiter=100, omega=0.5, phip=0.5, phig=0.5)
# print results
print('SVR hyperparameters:', xopt)
print('MAE:', fopt)
```
阅读全文
相关推荐
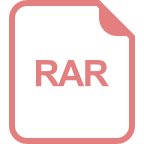
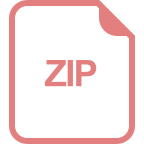
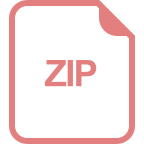















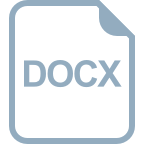