r如何提取文本的高频词
时间: 2024-05-13 18:14:38 浏览: 7
提取文本的高频词可以通过以下步骤:
1. 对文本进行分词处理,将文本按照词语进行划分。
2. 统计每个词语在文本中出现的频率。
3. 对词语按照频率进行排序,选择出现频率较高的词语即可。
具体实现可以使用Python中的NLTK或者Jieba等自然语言处理工具库。以下是一个示例代码:
```python
import jieba
from collections import Counter
# 打开文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 对文本进行分词处理
words = jieba.cut(text)
# 统计词语出现的频率
word_count = Counter(words)
# 按照词语频率排序,选择出现频率较高的前n个词
top_n = 10
top_words = word_count.most_common(top_n)
# 输出结果
for word, count in top_words:
print(word, count)
```
这段代码中,我们使用了Jieba库对文本进行了分词处理,并使用了Python标准库中的Counter类统计每个词语出现的频率。最后按照词语出现频率进行排序,选择出现频率较高的前n个词。
相关问题
jieba提取高频词
jieba是一个常用的中文分词工具,可以用于提取文本中的高频词。下面是使用jieba提取高频词的示例代码:
```python
import jieba
from collections import Counter
# 待分词的文本
text = "这是一个测试文本,用于演示jieba提取高频词的功能。"
# 使用jieba进行分词
words = jieba.cut(text)
# 统计词频
word_counts = Counter(words)
# 获取高频词
top_words = word_counts.most_common(5) # 提取前5个高频词
# 输出结果
for word, count in top_words:
print(word, count)
```
运行以上代码,将会输出文本中的前5个高频词及其出现次数。你也可以根据需要修改代码中的参数来提取不同数量的高频词。
python高频词提取
在Python中,你可以使用以下步骤来提取文本中的高频词:
1. 导入所需的库,如nltk、collections等。
2. 读取文本数据,并进行分词处理。可以使用nltk库中的word_tokenize()函数或自定义函数来完成。
3. 对分词后的结果进行去除停用词的操作。可以使用nltk库中的stopwords来完成。
4. 对去除停用词后的结果进行词频统计。可以使用collections库中的Counter函数来实现。
5. 对词频统计结果进行排序,以便查看高频词。可以使用sorted函数和lambda表达式来实现。
下面是一个示例代码,演示如何提取文本中的高频词:
```python
import nltk
from collections import Counter
from nltk.corpus import stopwords
nltk.download('stopwords')
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as file:
text = file.read()
# 分词处理
tokens = nltk.word_tokenize(text)
# 去除停用词
stop_words = set(stopwords.words('english'))
filtered_tokens = [token for token in tokens if token.lower() not in stop_words]
# 统计词频
word_count = Counter(filtered_tokens)
# 排序输出高频词
top_words = sorted(word_count.items(), key=lambda x: x[1], reverse=True)[:10]
print(top_words)
```
这段代码将输出文本中出现频率最高的10个单词。你可以根据需要调整代码中的参数,如停用词、输出数量等。
相关推荐
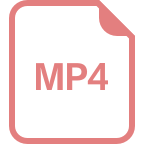
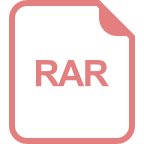
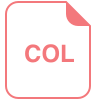
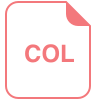
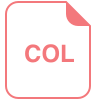









