cout << (c << 1) - (t * t == num)
时间: 2023-11-04 22:33:44 浏览: 98
This code snippet uses bitwise left shift operator (<<) to shift the value of variable c by 1 bit to the left, effectively multiplying it by 2. The result is then subtracted from a boolean expression (t * t == num) which evaluates to 1 or 0 depending on whether the square of variable t is equal to num.
The purpose of this code snippet is unclear without additional context, but it seems to be related to some calculation involving variables c, t, and num. The boolean expression may be used to conditionally adjust the result of the left shift operation.
相关问题
#include"Student.h" #include<iostream> #include<string> #include<fstream> using namespace std; Student::Student(int num, char* n, char* g, int m, int e, int c) { this->num = num; strcpy_s(name,n); strcpy_s(gender,g); Math = m; English = e; Computer = c; sum = Math + English + Computer; aver = sum / 3; } void Student::setNum(int num) { this->num = num; } void Student::setName(char* n) { strcpy_s(name,n); } void Student::setGender(char* g) { strcpy_s(gender,g); } void Student::setMath(int m) { Math = m; } void Student::setEnglish(int e) { English = e; } void Student::setComputer(int c) { Computer = c; } void Student::showStudentInfor() { cout << endl << "=========>> 显示学生成绩 <<=========" << endl; cout << "-------------------------------------------------------" << endl; cout << "学号" << "\t" << "姓名" << "\t" << "性别" << "\t" << "高数" << "\t" << "英语" << "\t" << "计算机" << "\t" << "平均分" << endl; cout << "-------------------------------------------------------" << endl; cout << num << "\t" << name << "\t" << gender << "\t" << Math << "\t" << English << "\t" << Computer << "\t" << aver << endl; }该怎样去定义且初始化Student类的对象数组
你可以使用以下方式定义并初始化一个名为students的Student对象数组:
```c++
Student students[3] = {
Student(1001, "张三", "男", 80, 75, 90),
Student(1002, "李四", "女", 85, 90, 87),
Student(1003, "王五", "男", 90, 88, 92)
};
```
这将创建一个长度为3的Student对象数组,每个对象都使用构造函数进行初始化。你可以根据需要更改数组的长度和对象的初始化值。注意,如果你的构造函数接受char*类型的参数,则必须使用strcpy_s函数将字符串复制到类的成员变量中,以避免内存错误。
#include <iostream> #include <ctime> using namespace std; struct student { int id; char gender; student* next; }; void twoQueue(student* hybrid, student*& girls, student*& boys); void output(student* girls, student* boys); void in(student* h, student* t); student* insert(student* head, student* t); int main() { int num; cin >> num; student* hybrid = NULL, * hybridCur = NULL, * tmp; for (int i = 0; i < num; i++) { tmp = new student; cin >> tmp->id >> tmp->gender; tmp->next = NULL; if (hybridCur == NULL) { hybridCur = tmp; hybrid = hybridCur; } else { hybridCur->next = tmp; hybridCur = hybridCur->next; } } student* girls = NULL, * boys = NULL; twoQueue(hybrid, girls, boys); output(girls, boys); } student* insert(student* head, student* t) { // 请在此添加代码,补全函数insertTail /********** Begin *********/ if (head == NULL) { t->next = NULL; return t; } student* cur = head; while (cur->next != NULL) { cur = cur->next; } cur->next = t; t->next = NULL; return head; /********** End **********/ } void in(student* h, student* t) { if (h == NULL) { t->next = NULL; h = t; } else { student* cur = h; while (cur->next) { cur = cur->next; } cur->next = t; t->next = NULL; } } void twoQueue(student* hybrid, student*& girls, student*& boys) { /**********Program**********/ student* cur = hybrid; student* pre = hybrid; student* g = girls; student* b = boys; int c = 0; while (cur) { if (cur->gender == 'F') { cur = cur->next; pre->next = NULL; in(girls, pre); c++; } else if (cur->gender == 'M') { cur = cur->next; pre->next = NULL; in(boys, pre); //cout << c << " " << pre->id << endl;// c++; } pre = cur; } /********** End **********/ } void output(student* girls, student* boys) { while (girls && girls->next) { cout << girls->id << ","; girls = girls->next; } if (girls) cout << girls->id << ";"; while (boys && boys->next) { cout << boys->id << ","; boys = boys->next; } if (boys) cout << boys->id << endl; }请帮我检查代码的错误
代码中存在一个问题,就是 `in` 函数对链表的头指针进行了修改,但是这个修改不会被传递到函数外部。因此,当 `in` 函数返回时,链表的头指针仍然是原来的值,没有被修改。
为了解决这个问题,`in` 函数的第一个参数应该是一个指向指针的指针(即 `student** h`),这样在函数内部就可以通过修改指针的值来改变链表头指针的值。
修改后的 `in` 函数代码如下:
```
void in(student** h, student* t) {
if (*h == NULL) {
t->next = NULL;
*h = t;
}
else {
student* cur = *h;
while (cur->next) {
cur = cur->next;
}
cur->next = t;
t->next = NULL;
}
}
```
另外,`insert` 函数没有被调用,可以将其删除。
阅读全文
相关推荐
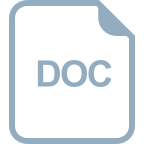
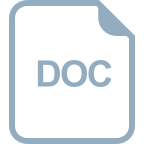
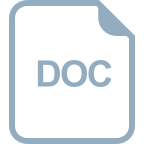














