在stm32f103中写一个函数,每按一下按键,返回变量加一
时间: 2024-12-13 11:24:38 浏览: 5
在STM32F103微控制器上编写一个函数,以便每当按下某个按键时,变量值递增,通常需要以下几个步骤:
1. **包含头文件**:首先,你需要包含相关的头文件,如`stm32f10x_gpio.h`、`stm32f10x_tim.h`(用于中断管理)和`stdbool.h`。
```c
#include "stm32f10x_gpio.h"
#include "stm32f10x_tim.h"
#include <stdbool.h>
```
2. **初始化GPIO和中断**:根据你的按键连接配置,设置GPIO为输入模式,并启用中断功能。例如,假设按键连接到PA0引脚:
```c
GPIO_InitTypeDef GPIO_InitStructure;
void EXTI_DeInit(void);
void EXTI_Init(void);
void EXTI_Pin_Configuration(void) {
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0; // PA0 for your button
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; // Input mode with pull-up
GPIO_Init(GPIOA, &GPIO_InitStructure);
EXTI_Init();
EXTI_Pin_Cmd(GPIO_Pin_0, ENABLE); // Enable the EXTI line for button detection
}
```
3. **初始化定时器**:这里我们可能使用一个定时器配合中断来检测按键触发。例如,可以使用TIM2定时器:
```c
void TIM2_IRQHandler() {
if (EXTI_GetITStatus(EXTI_Line0) != RESET) { // EXTI Line0 is associated with PA0
EXTI_ClearITPendingBit(EXTI_Line0);
increment_variable(); // Call the function to increment variable
}
}
void TIM2_Configuration() {
// Configure TIM2 for a specific period that allows triggering on key press
// ...
}
```
4. **定义变量和增加函数**:创建一个全局变量存储计数值,并编写一个函数处理每次按键触发:
```c
volatile uint8_t counter = 0;
void increment_variable() {
++counter;
}
```
5. **主程序**:在主循环中,配置中断并进入等待状态:
```c
int main(void) {
// Initialize GPIO, TIM2 and EXTI
EXTI_Pin_Configuration();
TIM2_Configuration();
// Enable global interrupt
NVIC_EnableIRQ(TIM2_IRQn);
while (1) {
// Main loop
}
}
```
6. **清除中断请求**:记得在中断服务函数中清除中断标志,防止无限循环。
现在,每当你按下连接到PA0的按键,`counter`变量就会递增。请注意,实际代码会根据你的硬件配置和需求调整细节。
阅读全文
相关推荐
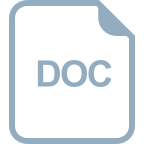
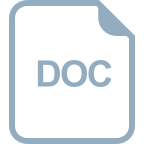
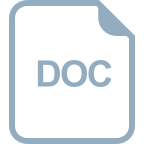



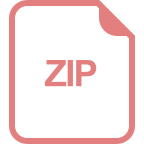











