ax.set_rgrids(np.arange(0.03, 3.5, 0.5), np.arange(-1, 2.5, 0.5), fontproperties="SimHei")
时间: 2023-10-23 10:08:56 浏览: 264
这段代码是用来设置极坐标图中半径轴的标签和网格线的。
`np.arange(0.03, 3.5, 0.5)` 生成了一个从0.03到3.5,步长为0.5的一维数组,用于设置半径轴的标签。
`np.arange(-1, 2.5, 0.5)` 生成了一个从-1到2.5,步长为0.5的一维数组,用于设置半径轴的网格线。
`fontproperties="SimHei"` 是设置标签字体为中文黑体。
`ax.set_rgrids()` 方法设置标签和网格线,第一个参数为半径轴标签的位置,第二个参数为半径轴网格线的位置,第三个参数为标签的字体。
相关问题
能帮我优化一下下面这段代码并增加一些注释吗import matplotlib matplotlib.use('Qt5Agg') from numpy import pi, sin import numpy as np import matplotlib.pyplot as plt from matplotlib.widgets import Slider, Button, RadioButtons def signal(amp, freq): return amp * sin(2 * pi * freq * t) axis_color = 'lightgoldenrodyellow' fig = plt.figure() ax = fig.add_subplot(111) fig.subplots_adjust(left=0.25, bottom=0.25) t = np.arange(-10, 10.0, 0.001) [line] = ax.plot(t, signal(5, 2), linewidth=2, color='red') ax.set_xlim([0, 1]) ax.set_ylim([-10, 10]) zoom_slider_ax = fig.add_axes([0.25, 0.1, 0.65, 0.03], facecolor=axis_color) zoom_slider = Slider(zoom_slider_ax, 'Zoom', -1, 1, valinit=0) def sliders_on_changed(val, scale_factor=0.25): cur_xlim = ax.get_xlim() cur_ylim = ax.get_ylim() scale = zoom_slider.val*scale_factor x_left = 0 + scale x_right = 1 - scale y_top = 10 - scale*10 y_bottom = -10 + scale*10 ax.set_xlim([x_left, x_right]) ax.set_ylim([y_bottom, y_top]) fig.canvas.draw_idle() zoom_slider.on_changed(sliders_on_changed) reset_button_ax = fig.add_axes([0.8, 0.025, 0.1, 0.04]) reset_button = Button(reset_button_ax, 'Reset', color=axis_color, hovercolor='0.975') def reset_button_on_clicked(mouse_event): zoom_slider.reset() reset_button.on_clicked(reset_button_on_clicked) color_radios_ax = fig.add_axes([0.025, 0.5, 0.15, 0.15], facecolor=axis_color) color_radios = RadioButtons(color_radios_ax, ('red', 'blue', 'green'), active=0) def color_radios_on_clicked(label): line.set_color(label) fig.canvas.draw_idle() color_radios.on_clicked(color_radios_on_clicked) plt.show()
当然可以,下面是代码的优化和注释:
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.widgets import Slider, Button, RadioButtons
# 定义信号函数
def signal(amp, freq, t):
return amp * np.sin(2 * np.pi * freq * t)
# 设置图形参数
axis_color = 'lightgoldenrodyellow'
fig, ax = plt.subplots()
fig.subplots_adjust(left=0.25, bottom=0.25)
# 设置时间轴
t = np.arange(-10, 10.0, 0.001)
# 绘制信号曲线
[line] = ax.plot(t, signal(5, 2, t), linewidth=2, color='red')
ax.set_xlim([0, 1])
ax.set_ylim([-10, 10])
# 添加缩放滑块
zoom_slider_ax = fig.add_axes([0.25, 0.1, 0.65, 0.03], facecolor=axis_color)
zoom_slider = Slider(zoom_slider_ax, 'Zoom', -1, 1, valinit=0)
# 滑块改变时的回调函数
def sliders_on_changed(val, scale_factor=0.25):
cur_xlim = ax.get_xlim()
cur_ylim = ax.get_ylim()
scale = zoom_slider.val*scale_factor
x_left = 0 + scale
x_right = 1 - scale
y_top = 10 - scale*10
y_bottom = -10 + scale*10
ax.set_xlim([x_left, x_right])
ax.set_ylim([y_bottom, y_top])
fig.canvas.draw_idle()
zoom_slider.on_changed(sliders_on_changed)
# 添加重置按钮
reset_button_ax = fig.add_axes([0.8, 0.025, 0.1, 0.04])
reset_button = Button(reset_button_ax, 'Reset', color=axis_color, hovercolor='0.975')
# 重置按钮点击时的回调函数
def reset_button_on_clicked(mouse_event):
zoom_slider.reset()
reset_button.on_clicked(reset_button_on_clicked)
# 添加颜色选择按钮
color_radios_ax = fig.add_axes([0.025, 0.5, 0.15, 0.15], facecolor=axis_color)
color_radios = RadioButtons(color_radios_ax, ('red', 'blue', 'green'), active=0)
# 颜色选择按钮点击时的回调函数
def color_radios_on_clicked(label):
line.set_color(label)
fig.canvas.draw_idle()
color_radios.on_clicked(color_radios_on_clicked)
plt.show()
```
优化如下:
1. 将 `sin` 改为 `np.sin`,避免使用 `from numpy import *` 导致命名冲突或覆盖内置函数。
2. 将 `signal` 函数中的 `t` 参数移动到函数参数列表中,增加代码可读性。
3. 将 `fig, ax` 和 `plt.subplots()` 合并成一个语句,简化代码。
4. 在 `Slider`、`Button` 和 `RadioButtons` 的初始化中,添加 `color` 和 `hovercolor` 参数,避免鼠标悬停时颜色过于明亮。
5. 将回调函数和控件的绑定分别放在不同的代码块中,增加代码可读性。
\begin{bmatrix}u \\v\end{bmatrix}=\begin{bmatrix} \cos\theta & \sin\theta \\-\sin \theta &\cos\theta \end{bmatrix} \times \left \{ \begin{bmatrix}k _{x} -1 &\gamma _{x } \\\gamma _{y } &k _{y} -1\end{bmatrix}\times \begin{bmatrix} x\\y\end{bmatrix} +{\textstyle \sum_{j=1}^{N}\begin{bmatrix}A_{y}^{j} e^{\frac{-1}{2} \left ( \frac{y-y_{1}^{j} }{\sigma _{y_{1} }^{j} } \right )^2-\frac{1}{2} \left (\frac{x-x_{1}^{j} }{\sigma _{x_{1} }^{j} } \right ) ^2 } \\A_{x}^{j} e^{\frac{-1}{2} \left ( \frac{x-x_{0}^{j} }{\sigma _{x_{0} }^{j} } \right )^2-\frac{1}{2} \left (\frac{y-y_{0}^{j} }{\sigma _{y_{0} }^{j} } \right ) ^2 }\end{bmatrix}} \right \} +\begin{bmatrix}t_{x} \\t_{y}\end{bmatrix},N=1or2 X=y=256,tx,ty 范围:-4.0到4.0像素,有效最大位移:2.0像素;kx,ky 范围:0.96到1.04,有效最大位移:5.1;theta 范围:-0.01至0.01rad , 有效最大位移: 2.4像素;gammax,gammay 范围:-0.03至0.03,有效最大位移: 3.8像素;Ax,Ay范围:0.003到0.6 ;sigmaх0,sigmay0,sigmax1,sigmay1范围:0.06到0.5,x0,y0,x1,y1范围:0到511,最终生成一个可视化的应边场图片(w.r.t最终图像大小:128x128 )
根据您提供的方程和参数范围,以下是一个更新后的代码示例,用于生成可视化的应边场图片:
```python
import numpy as np
import matplotlib.pyplot as plt
# 参数设置
N = np.random.choice([1, 2]) # 随机选择N的值为1或2
X = y = 256 # 图像大小为256x256像素
tx_min, tx_max = -4.0, 4.0
ty_min, ty_max = -4.0, 4.0
kx_min, kx_max = 0.96, 1.04
ky_min, ky_max = 0.96, 1.04
theta_min, theta_max = -0.01, 0.01
gammax_min, gammax_max = -0.03, 0.03
gammay_min, gammay_max = -0.03, 0.03
Ax_min, Ax_max = 0.003, 0.6
Ay_min, Ay_max = 0.003, 0.6
sigmax0_min, sigmax0_max = 0.06, 0.5
sigmay0_min, sigmay0_max = 0.06, 0.5
sigmax1_min, sigmax1_max = 0.06, 0.5
sigmay1_min, sigmay1_max = 0.06, 0.5
x0_min, x0_max = 0, 511
y0_min, y0_max = 0, 511
x1_min, x1_max = 0, 511
y1_min, y1_max = 0, 511
# 随机生成参数值
tx = np.random.uniform(tx_min, tx_max)
ty = np.random.uniform(ty_min, ty_max)
kx = np.random.uniform(kx_min, kx_max)
ky = np.random.uniform(ky_min, ky_max)
theta = np.random.uniform(theta_min, theta_max)
gammax = np.random.uniform(gammax_min, gammax_max)
gammay = np.random.uniform(gammay_min, gammay_max)
Ax = np.random.uniform(Ax_min, Ax_max)
Ay = np.random.uniform(Ay_min, Ay_max)
sigmax0 = np.random.uniform(sigmax0_min, sigmax0_max)
sigmay0 = np.random.uniform(sigmay0_min, sigmay0_max)
sigmax1 = np.random.uniform(sigmax1_min, sigmax1_max)
sigmay1 = np.random.uniform(sigmay1_min, sigmay1_max)
x0 = np.random.randint(x0_min, x0_max+1)
y0 = np.random.randint(y0_min, y0_max+1)
x1 = np.random.randint(x1_min, x1_max+1)
y1 = np.random.randint(y1_min, y1_max+1)
# 生成网格坐标
x_grid, y_grid = np.meshgrid(range(X), range(y))
# 计算数学方程
A = np.array([[kx-1, gammay], [gammax, ky-1]])
B = np.stack((x_grid, y_grid), axis=-1).reshape(-1, 2).T
C = np.array([[Ay * np.exp(-0.5 * ((y_grid-y1)/sigmay1)**2 - 0.5 * ((x_grid-x1)/sigmax1)**2)],
[Ax * np.exp(-0.5 * ((x_grid-x0)/sigmax0)**2 - 0.5 * ((y_grid-y0)/sigmay0)**2)]])
D = np.dot(A, np.dot(B, C)) + np.array([[tx], [ty]])
u = D[0].reshape(X, y)
v = D[1].reshape(X, y)
# 绘制图像
fig, ax = plt.subplots(figsize=(5, 5))
plt.quiver(x_grid, y_grid, u, v)
ax.set_xlim(0, X-1)
ax.set_ylim(0, y-1)
plt.grid(True)
plt.show()
```
这段代码使用了`np.meshgrid`函数生成网格坐标,并计算出每个坐标点对应的u和v值。然后使用`plt.quiver`函数绘制出应边场图片。最终生成的图片大小为128x128像素,范围在0到255之间。你可以根据需要自行调整参数范围和图像大小。
阅读全文
相关推荐
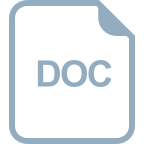
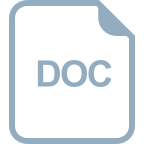
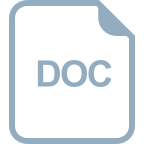
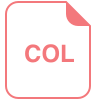

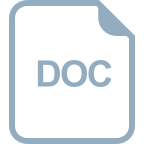
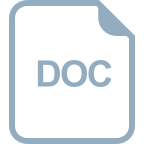
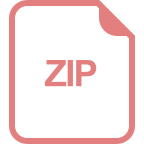
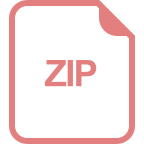
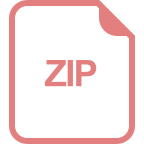
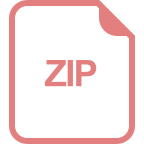
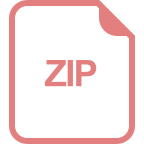
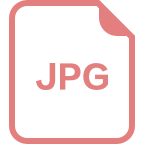
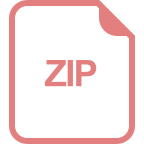