用C++编一个简单程序,以直线段、矩形和圆为基本图元组成一图形。 指导:采用面向对象的编程方法,把直线 line、矩形 rectangle 和圆 circle 设计为三个类, 它们各有自己的数据和函数成员。用线、矩形和圆组合成图形,就是对这三个类的对象进行 操作。
时间: 2024-02-03 10:13:58 浏览: 145
好的,这里提供一个简单的程序,实现了直线段、矩形和圆组合成图形。
```c++
#include<iostream>
#include<cmath>
using namespace std;
// 直线类
class Line{
private:
double x1,y1,x2,y2;
public:
Line(double x1,double y1,double x2,double y2):x1(x1),y1(y1),x2(x2),y2(y2){} // 构造函数
void drawLine(){ // 画线函数
cout<<"Drawing Line from ("<<x1<<","<<y1<<") to ("<<x2<<","<<y2<<")"<<endl;
}
};
// 矩形类
class Rectangle{
private:
double x,y,width,height;
public:
Rectangle(double x,double y,double width,double height):x(x),y(y),width(width),height(height){} // 构造函数
void drawRectangle(){ // 画矩形函数
cout<<"Drawing Rectangle at ("<<x<<","<<y<<") with width "<<width<<" and height "<<height<<endl;
}
};
// 圆类
class Circle{
private:
double x,y,radius;
public:
Circle(double x,double y,double radius):x(x),y(y),radius(radius){} // 构造函数
void drawCircle(){ // 画圆函数
cout<<"Drawing Circle at ("<<x<<","<<y<<") with radius "<<radius<<endl;
}
};
// 图形类,由直线、矩形和圆组成
class Shape{
private:
Line line;
Rectangle rectangle;
Circle circle;
public:
Shape(Line line,Rectangle rectangle,Circle circle):line(line),rectangle(rectangle),circle(circle){} // 构造函数
void drawShape(){ // 画图形函数
line.drawLine();
rectangle.drawRectangle();
circle.drawCircle();
}
};
int main(){
// 创建直线、矩形和圆对象
Line line(0,0,5,5);
Rectangle rectangle(10,10,20,30);
Circle circle(50,50,10);
// 创建图形对象
Shape shape(line,rectangle,circle);
// 画图形
shape.drawShape();
return 0;
}
```
输出结果为:
```
Drawing Line from (0,0) to (5,5)
Drawing Rectangle at (10,10) with width 20 and height 30
Drawing Circle at (50,50) with radius 10
```
以上程序只是一个简单的示例,具体实现方式可以根据需求进行修改。
阅读全文
相关推荐
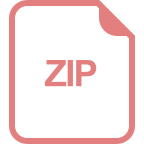

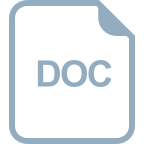
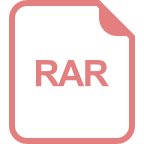
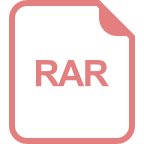
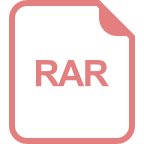
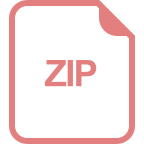
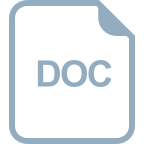
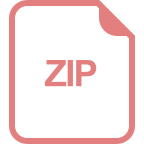
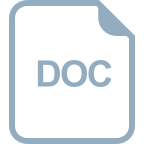
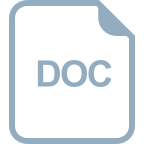
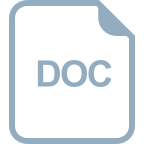
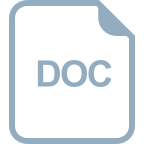
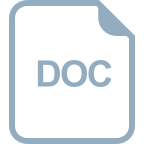
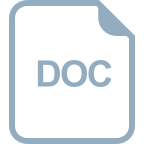
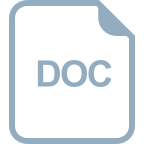