Tkinter编写一个程序显示递归树且可控制分叉数且只在枝头分叉
时间: 2024-05-07 15:20:13 浏览: 91
下面是一个简单的Tkinter程序,可以显示递归树并且可以控制分叉数,只在枝头分叉。代码中使用了递归的方式生成树形结构,并使用Canvas来显示。
```python
import tkinter as tk
class Tree:
def __init__(self, canvas, x, y, angle, depth, max_depth, branch_len, branch_width, branch_angle):
self.canvas = canvas
self.x = x
self.y = y
self.angle = angle
self.depth = depth
self.max_depth = max_depth
self.branch_len = branch_len
self.branch_width = branch_width
self.branch_angle = branch_angle
def draw(self):
if self.depth > self.max_depth:
return
x2 = self.x + self.branch_len * tk.cos(self.angle)
y2 = self.y - self.branch_len * tk.sin(self.angle)
self.canvas.create_line(self.x, self.y, x2, y2, width=self.branch_width)
self.angle += self.branch_angle
if self.depth < self.max_depth:
for i in range(self.branch_width):
Tree(self.canvas, x2, y2, self.angle, self.depth + 1, self.max_depth,
self.branch_len * 0.8, self.branch_width - 1, self.branch_angle * 0.8).draw()
def set_branch_width(self, width):
self.branch_width = width
def set_branch_angle(self, angle):
self.branch_angle = angle
class App:
def __init__(self):
self.window = tk.Tk()
self.window.title("Recursive Tree")
self.canvas = tk.Canvas(self.window, width=600, height=600, bg='white')
self.canvas.pack()
self.depth_scale = tk.Scale(self.window, from_=1, to=10, orient=tk.HORIZONTAL, label='Depth',
command=self.draw_tree)
self.depth_scale.pack()
self.branch_scale = tk.Scale(self.window, from_=10, to=100, orient=tk.HORIZONTAL, label='Branch Length',
command=self.draw_tree)
self.branch_scale.pack()
self.width_scale = tk.Scale(self.window, from_=1, to=10, orient=tk.HORIZONTAL, label='Branch Width',
command=self.set_branch_width)
self.width_scale.pack()
self.angle_scale = tk.Scale(self.window, from_=0, to_=360, orient=tk.HORIZONTAL, label='Branch Angle',
command=self.set_branch_angle)
self.angle_scale.pack()
self.draw_tree()
self.window.mainloop()
def draw_tree(self, event=None):
self.canvas.delete('all')
max_depth = self.depth_scale.get()
branch_len = self.branch_scale.get()
branch_width = self.width_scale.get()
branch_angle = self.angle_scale.get() * tk.pi / 180
Tree(self.canvas, 300, 500, -tk.pi / 2, 1, max_depth, branch_len, branch_width, branch_angle).draw()
def set_branch_width(self, width):
self.canvas.delete('all')
max_depth = self.depth_scale.get()
branch_len = self.branch_scale.get()
branch_width = int(width)
branch_angle = self.angle_scale.get() * tk.pi / 180
tree = Tree(self.canvas, 300, 500, -tk.pi / 2, 1, max_depth, branch_len, branch_width, branch_angle)
tree.draw()
tree.set_branch_width(branch_width)
def set_branch_angle(self, angle):
self.canvas.delete('all')
max_depth = self.depth_scale.get()
branch_len = self.branch_scale.get()
branch_width = self.width_scale.get()
branch_angle = int(angle) * tk.pi / 180
tree = Tree(self.canvas, 300, 500, -tk.pi / 2, 1, max_depth, branch_len, branch_width, branch_angle)
tree.draw()
tree.set_branch_angle(branch_angle)
if __name__ == '__main__':
app = App()
```
程序中使用了Scale控件来控制树的深度、分支长度、分支宽度和分支角度。在程序中,我们使用Tree类来表示树的结构,并使用draw方法来递归绘制树的结构。set_branch_width和set_branch_angle方法可以用来改变分支的宽度和角度。
阅读全文
相关推荐
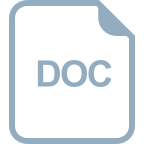
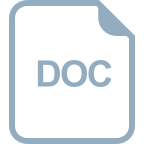
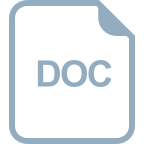
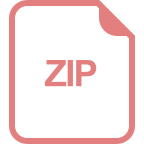
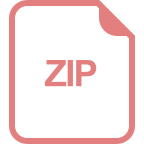
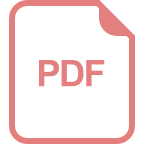
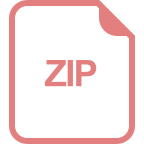
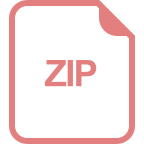
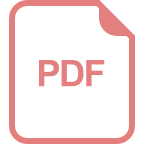
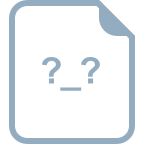
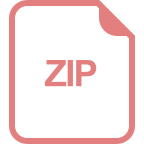
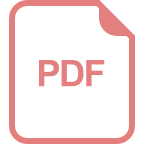
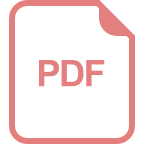
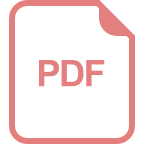
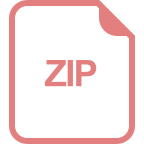
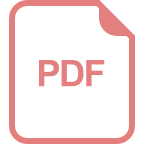