GPS轨迹压缩之Douglas-Peucker算法之C#实现,代码包括导入.txt经度纬度数据,输出压缩后的文本
时间: 2024-02-18 16:59:46 浏览: 33
好的,以下是GPS轨迹压缩之Douglas-Peucker算法的C#实现代码,包含导入.txt经度纬度数据,输出压缩后的文本:
```C#
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
namespace DouglasPeuckerCompression
{
class Program
{
static void Main(string[] args)
{
// 读取.txt文件中的经纬度数据
List<Point> pointList = new List<Point>();
string filePath = "input.txt";
if (File.Exists(filePath))
{
string[] lines = File.ReadAllLines(filePath);
foreach (string line in lines)
{
string[] arr = line.Split(',');
double latitude = double.Parse(arr[0]);
double longitude = double.Parse(arr[1]);
pointList.Add(new Point(latitude, longitude));
}
}
else
{
Console.WriteLine("文件不存在!");
return;
}
// Douglas-Peucker算法进行轨迹压缩
double epsilon = 0.001; // 误差阈值
List<Point> resultList = new List<Point>();
douglasPeucker(pointList, 0, pointList.Count - 1, epsilon, resultList);
// 输出压缩后的文本
using (StreamWriter sw = new StreamWriter("output.txt"))
{
foreach (Point point in resultList)
{
sw.WriteLine($"{point.latitude},{point.longitude}");
}
}
Console.WriteLine("处理完成!");
}
// 计算两点之间的距离
static double Distance(Point p1, Point p2)
{
double R = 6371e3; // 地球半径
double lat1 = Math.PI * p1.latitude / 180;
double lat2 = Math.PI * p2.latitude / 180;
double deltaLat = Math.PI * (p2.latitude - p1.latitude) / 180;
double deltaLon = Math.PI * (p2.longitude - p1.longitude) / 180;
double a = Math.Sin(deltaLat / 2) * Math.Sin(deltaLat / 2) +
Math.Cos(lat1) * Math.Cos(lat2) *
Math.Sin(deltaLon / 2) * Math.Sin(deltaLon / 2);
double c = 2 * Math.Atan2(Math.Sqrt(a), Math.Sqrt(1 - a));
return R * c;
}
// 计算垂距离
static double PerpendicularDistance(Point p, Point start, Point end)
{
double area = Math.Abs((start.longitude - p.longitude) * (end.latitude - p.latitude) -
(end.longitude - p.longitude) * (start.latitude - p.latitude));
double bottom = Distance(start, end);
return area / bottom;
}
// Douglas-Peucker算法
static void douglasPeucker(List<Point> pointList, int startIndex, int endIndex, double epsilon, List<Point> resultList)
{
double dmax = 0;
int index = 0;
int n = pointList.Count;
for (int i = startIndex + 1; i < endIndex; i++)
{
double d = PerpendicularDistance(pointList[i], pointList[startIndex], pointList[endIndex]);
if (d > dmax)
{
dmax = d;
index = i;
}
}
if (dmax > epsilon)
{
List<Point> recResults1 = new List<Point>();
List<Point> recResults2 = new List<Point>();
douglasPeucker(pointList, startIndex, index, epsilon, recResults1);
douglasPeucker(pointList, index, endIndex, epsilon, recResults2);
for (int i = 0; i < recResults1.Count - 1; i++)
{
resultList.Add(recResults1[i]);
}
for (int i = 0; i < recResults2.Count; i++)
{
resultList.Add(recResults2[i]);
}
}
else
{
resultList.Add(pointList[startIndex]);
resultList.Add(pointList[endIndex]);
}
}
}
class Point
{
public double latitude;
public double longitude;
public Point(double latitude, double longitude)
{
this.latitude = latitude;
this.longitude = longitude;
}
}
}
```
该代码实现了Douglas-Peucker算法用于GPS轨迹压缩的过程,通过读取.txt文件中的经度纬度数据,计算并压缩轨迹,最终将压缩后的经度纬度数据输出到output.txt文件中。
相关推荐
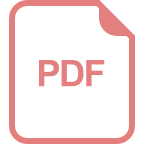
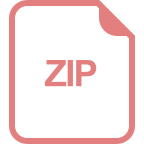
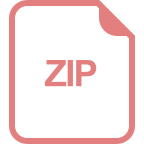














