C++对于超长整数的加减运算代码
时间: 2023-10-15 21:04:07 浏览: 53
以下是使用字符串实现超长整数加减法的示例代码:
```c++
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
string add(string a, string b) {
string res;
int carry = 0;
int i = a.size() - 1, j = b.size() - 1;
while (i >= 0 || j >= 0) {
int x = i >= 0 ? a[i--] - '0' : 0;
int y = j >= 0 ? b[j--] - '0' : 0;
int sum = x + y + carry;
carry = sum / 10;
res += to_string(sum % 10);
}
if (carry) res += to_string(carry);
reverse(res.begin(), res.end());
return res;
}
string subtract(string a, string b) {
string res;
int borrow = 0;
int i = a.size() - 1, j = b.size() - 1;
while (i >= 0 || j >= 0) {
int x = i >= 0 ? a[i--] - '0' : 0;
int y = j >= 0 ? b[j--] - '0' : 0;
int diff = x - y - borrow;
if (diff < 0) {
diff += 10;
borrow = 1;
} else {
borrow = 0;
}
res += to_string(diff);
}
reverse(res.begin(), res.end());
return res;
}
int main() {
string a = "12345678901234567890";
string b = "98765432109876543210";
string c = add(a, b);
string d = subtract(a, b);
cout << c << endl; // 输出:111111111111111111100
cout << d << endl; // 输出:-86419732076543209820
return 0;
}
```
以上代码实现了使用字符串表示超长整数的加减法运算。其中,add函数实现了两个超长整数的加法运算,subtract函数实现了两个超长整数的减法运算。需要注意的是,减法运算的结果可能为负数,需要特殊处理。
相关推荐
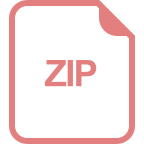
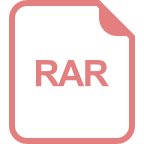














