1. 定义单链表的结点以及指针结构。 2. 采用头插入法和尾插入法构建单链表。 3. 遍历单链表并输出每个结点的数据值。 4. 实现单链表的按位查找和按值查找操作。 5. 实现单链表的位插入和删除操作
时间: 2024-10-24 18:07:24 浏览: 67
1. 单链表是一种线性数据结构,每个节点由两个部分组成:数据域(存放数据)和指针域(指向下一个节点的地址)。在最简单的形式中,一个链表节点可以定义为:
```cpp
struct ListNode {
int data; // 存放数据的部分
ListNode* next; // 指向下一个节点的指针,初始为空(NULL)
};
```
2. - **头插入法**:新节点作为头结点插入,将新节点的next指向当前头结点,然后更新头指针。
```cpp
void insertAtHead(ListNode** head, int value) {
ListNode* newNode = new ListNode{value, *head};
*head = newNode;
}
```
- **尾插入法**:从最后一个节点开始向前查找,找到最后一个节点并将其next指向下新节点,再创建新节点。
```cpp
void insertAtTail(ListNode** head, int value) {
if (*head == nullptr) {
*head = new ListNode{value, nullptr};
return;
}
ListNode* current = *head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new ListNode{value, nullptr};
}
```
3. 遍历单链表并输出每个节点的数据,通常使用递归或循环结构:
```cpp
void printList(ListNode* head) {
if (head == nullptr) {
return;
}
std::cout << head->data << " ";
printList(head->next);
}
```
4. **按位查找**:链表本身并不支持高效的位查找,因为它是连续存储的,不适合随机访问。如果是整数列表,并希望高效地查找某个位是否为1,应该转换为数组或其他适合的结构。
**按值查找**:在链表中查找特定值,需要逐个节点对比:
```cpp
bool search(ListNode* head, int target) {
ListNode* current = head;
while (current != nullptr) {
if (current->data == target) {
return true;
}
current = current->next;
}
return false;
}
```
5. **位插入**:如要在指定位置插入元素,需先定位到该位置,然后插入新节点:
```cpp
void insertAtPosition(ListNode** head, int value, int position) {
if (position <= 0) {
insertAtHead(head, value);
} else {
ListNode* current = *head;
for (int i = 0; i < position - 1 && current != nullptr; ++i) {
current = current->next;
}
if (current != nullptr) {
ListNode* newNode = new ListNode{value, current->next};
current->next = newNode;
}
}
}
void deleteByValue(ListNode** head, int value) {
ListNode* prev = nullptr, *current = *head;
while (current != nullptr) {
if (current->data == value) {
if (prev == nullptr) {
*head = current->next;
} else {
prev->next = current->next;
}
delete current;
break;
}
prev = current;
current = current->next;
}
}
```
阅读全文
相关推荐
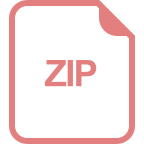
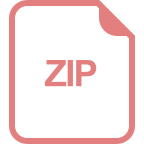
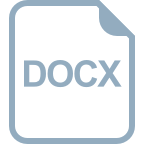

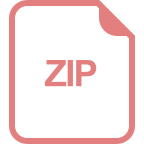
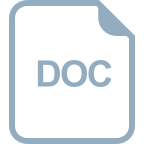
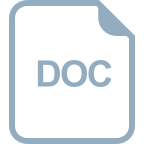
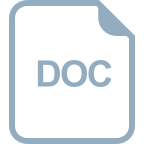
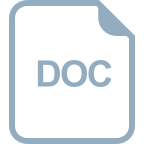









