android rgba byte[] 转 Bitmap
时间: 2023-12-07 17:05:55 浏览: 40
可以使用BitmapFactory类中的decodeByteArray方法将RGBA格式的byte数组转换为Bitmap,具体实现代码如下:
```java
public Bitmap rgbaByteArrayToBitmap(byte[] data, int width, int height) {
Bitmap bitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
ByteBuffer buffer = ByteBuffer.wrap(data);
bitmap.copyPixelsFromBuffer(buffer);
return bitmap;
}
```
其中,data为RGBA格式的byte数组,width和height分别为图片的宽度和高度。通过调用Bitmap.createBitmap方法创建一个空的Bitmap对象,然后通过ByteBuffer.wrap方法将data包装为ByteBuffer,最后调用Bitmap.copyPixelsFromBuffer方法将数据复制到Bitmap对象中。
相关问题
android RGBA转bitmap
下面是一段将 RGBA 转换为 Bitmap 的示例代码:
```java
public Bitmap rgbaToBitmap(byte[] pixels, int width, int height) {
Bitmap bitmap = Bitmap.createBitmap(width, height, Bitmap.Config.ARGB_8888);
ByteBuffer buffer = ByteBuffer.wrap(pixels);
bitmap.copyPixelsFromBuffer(buffer);
return bitmap;
}
```
其中,pixels 是一个 byte 数组,表示每个像素点的 RGBA 值,width 和 height 分别表示图片的宽度和高度。这段代码创建了一个 ARGB_8888 类型的 Bitmap 对象,并将 byte 数组中的 RGBA 值转换为 ARGB 值,最后将其设置为 Bitmap 的像素点。这里使用了 ByteBuffer 类型来读取 byte 数组中的数据,并将其拷贝到 Bitmap 中。最后返回生成的 Bitmap 对象。
bitmap 转opencv MAt
Bitmap 是一种在 Android 平台上使用的图像存储格式,而 OpenCV 是一种广泛使用的计算机视觉库。如果你想将 Bitmap 转换为 OpenCV 的 Mat 格式,可以按照以下步骤进行:
1. 首先,获取 Bitmap 的像素数据。可以使用 Bitmap 的 getPixels() 方法将像素数据保存到一个 int 数组中。
2. 然后,创建一个新的 Mat 对象,指定其宽度、高度和像素类型。可以使用 CV_8UC4 类型来存储 Bitmap 的像素数据。
3. 将 int 数组中的像素数据复制到新的 Mat 对象中,使用 memcpy() 函数。
以下是示例代码:
```
// 获取 Bitmap 的像素数据
int[] pixels = new int[bitmap.getWidth() * bitmap.getHeight()];
bitmap.getPixels(pixels, 0, bitmap.getWidth(), 0, 0, bitmap.getWidth(), bitmap.getHeight());
// 创建新的 Mat 对象
Mat mat = new Mat(bitmap.getHeight(), bitmap.getWidth(), CvType.CV_8UC4);
// 将像素数据复制到 Mat 对象中
byte[] rgba = new byte;
for (int i = 0; i < pixels.length; i++) {
int p = pixels[i];
rgba = (byte) Color.red(p);
rgba = (byte) Color.green(p);
rgba = (byte) Color.blue(p);
rgba = (byte) Color.alpha(p);
mat.put(i / mat.cols(), i % mat.cols(), rgba);
}
```
相关推荐
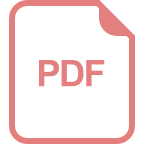
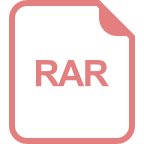










