MFC中使用贝塞尔曲线绘制的椭圆在图像上,怎么实现椭圆随图像旋转
时间: 2024-03-27 13:40:46 浏览: 25
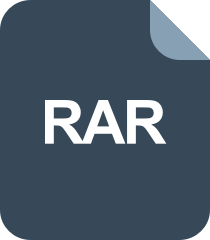
在MFC下实现图像的缩放和旋转

要实现椭圆随图像旋转,可以使用MFC提供的GDI+库中的旋转变换函数。具体步骤如下:
1. 绘制椭圆:使用贝塞尔曲线的二次曲线段绘制椭圆,与之前的方法相同。
2. 计算旋转角度:可以通过图像变换库中的`Graphics::GetLastStatus()`函数来判断变换是否成功,然后使用`Graphics::RotateTransform()`函数对图像进行旋转。
3. 应用旋转变换:使用`Graphics::DrawBezier()`函数来绘制椭圆,同时应用旋转变换。
以下是一个简单的示例代码,可以实现椭圆随图像旋转:
```C++
// 绘制椭圆
void DrawEllipse(Graphics* pGraphics, const PointF& center, float rx, float ry)
{
const int n = 100; // 曲线段数
const float dt = 2 * PI / n; // 每段曲线的弧度值
PointF pts[4 * n]; // 存储曲线端点
for (int i = 0; i < n; i++) {
float t = i * dt;
float x = rx * cos(t);
float y = ry * sin(t);
pts[4 * i] = PointF(center.X + x, center.Y + y);
pts[4 * i + 1] = PointF(center.X + x, center.Y - y);
pts[4 * i + 2] = PointF(center.X - x, center.Y - y);
pts[4 * i + 3] = PointF(center.X - x, center.Y + y);
}
pGraphics->DrawBezier(&Pen(Color::Black), pts, 4 * n - 1);
}
// 旋转椭圆
void RotateEllipse(Graphics* pGraphics, const PointF& center, float rx, float ry, float angle)
{
pGraphics->TranslateTransform(center.X, center.Y); // 将坐标系移到椭圆中心点
pGraphics->RotateTransform(angle); // 旋转坐标系
DrawEllipse(pGraphics, PointF(0, 0), rx, ry); // 绘制椭圆
}
// 绘制图像
void DrawImage(Graphics* pGraphics, Image* pImage, float x, float y, float angle)
{
Status status = pGraphics->GetLastStatus();
if (status == Ok) {
pGraphics->TranslateTransform(x, y); // 将坐标系移到图像中心点
pGraphics->RotateTransform(angle); // 旋转坐标系
pGraphics->DrawImage(pImage, -pImage->GetWidth() / 2, -pImage->GetHeight() / 2); // 绘制图像
}
}
// 绘制图像和椭圆
void DrawImageAndEllipse(Graphics* pGraphics, Image* pImage, const PointF& center, float rx, float ry, float angle)
{
DrawImage(pGraphics, pImage, center.X, center.Y, angle); // 绘制图像
RotateEllipse(pGraphics, center, rx, ry, angle); // 旋转椭圆
}
```
在使用时,可以先调用`DrawImageAndEllipse`函数绘制图像和椭圆,然后再调用`DrawImage`函数旋转图像即可。
阅读全文
相关推荐
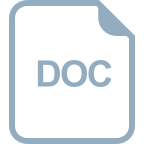
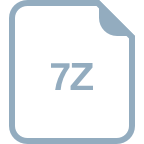






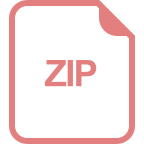
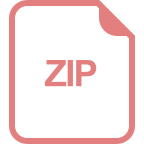
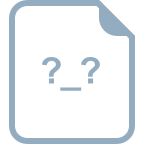
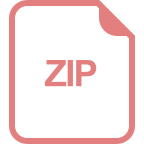
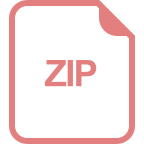
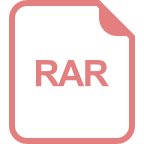
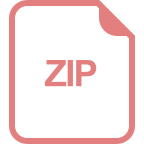