edges = cv2.Canny(binary, 30, 150)
时间: 2024-04-06 09:31:29 浏览: 233
这段代码使用OpenCV库中的Canny函数对二值化图像进行边缘检测。Canny函数的第一个参数是待处理的二值化图像,第二个参数是阈值1,第三个参数是阈值2,用于控制边缘的检测结果。具体来说,对于图像中的每一个像素,如果其梯度值大于阈值2,则被标记为强边缘;如果其梯度值小于阈值1,则被标记为非边缘;如果其梯度值介于阈值1和阈值2之间,则根据其是否与强边缘相连来判断其是否为边缘。最终,该函数返回一个二值化的边缘图像,其中边缘部分为白色,非边缘部分为黑色。
相关问题
将下述代码增加显示边缘图像功能: import cv2 import numpy as np from dxfwrite import DXFEngine as dxf img = cv2.imread('ft.jpg', 0) edges = cv2.Canny(img, 100, 200) thresh = cv2.threshold(edges, 27, 255, cv2.THRESH_BINARY)[1] contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) dwg = dxf.drawing('output2.dxf') for contour in contours: if len(contour) > 1: dwg.add(dxf.polyline(contour[:, 0, :])) dwg.save()
可以在代码最后添加以下代码来显示边缘图像:
```
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
完整代码如下:
```
import cv2
import numpy as np
from dxfwrite import DXFEngine as dxf
img = cv2.imread('ft.jpg', 0)
edges = cv2.Canny(img, 100, 200)
thresh = cv2.threshold(edges, 27, 255, cv2.THRESH_BINARY)[1]
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
dwg = dxf.drawing('output2.dxf')
for contour in contours:
if len(contour) > 1:
dwg.add(dxf.polyline(contour[:, 0, :]))
dwg.save()
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
运行代码后,会在生成的 DXF 文件同时显示边缘图像。
img = cv2.imread('../maze3.png') # 灰度化、阈值分割、边缘检测 gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY) edges = cv2.Canny(thresh, 50, 150, apertureSize=3) # 霍夫变换检测直线 lines = cv2.HoughLines(edges, 1, np.pi/180, 100) # 绘制直线 if lines is not None and len(lines) > 0: for line in lines: rho, theta = line[0] a = np.cos(theta) b = np.sin(theta) x0 = a * rho y0 = b * rho x1 = int(x0 + 1000 * (-b)) y1 = int(y0 + 1000 * (a)) x2 = int(x0 - 1000 * (-b)) y2 = int(y0 - 1000 * (a)) # 判断直线是否在黑色区域内 if thresh[y1, x1] == 0 and thresh[y2, x2] == 0: cv2.line(img, (x1, y1), (x2, y2), (0, 0, 0), 2)
这段代码是对一张图片进行灰度化、阈值分割、边缘检测、霍夫变换检测直线、绘制直线的过程。在绘制直线的时候,会判断直线是否在黑色区域内,如果不在,则不绘制这条直线。
错误信息提示说 IndexError: index -1000 is out of bounds for axis 1 with size 588,说明在使用 thresh[y1, x1] 和 thresh[y2, x2] 进行判断时,出现了数组越界的问题,即坐标值超过了 thresh 数组的范围。
你可以尝试在判断之前,打印出 x1, y1, x2, y2 这四个值,看看是否有异常,以及其具体的数值。如果坐标值确实超出了范围,那么你可以检查一下代码中的变量,看看是否有误。另外,也可以检查一下原始图片的尺寸,是否与代码中的处理一致。
阅读全文
相关推荐
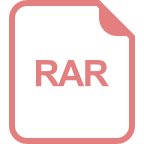













