import cv2 import glob import numpy as np imgs = glob.glob("maze.png") res, L, N = [], 256, 5 for i in imgs: img = cv2.imread(i) img = cv2.resize(img, (512, 512)) gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) _, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY_INV) # contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) max_contour = max(contours, key=cv2.contourArea) epsilon = 0.1 * cv2.arcLength(max_contour, True) approx = cv2.approxPolyDP(max_contour, epsilon, True) circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT, 1, 20, param1=50, param2=15, minRadius=5, maxRadius=15) if circles is not None: circles = np.round(circles[0, :]).astype("int") for (x, y, r) in circles: cv2.circle(img, (x, y), r, (0, 0, 255), 2) # edges = cv2.Canny(gray, 100, 200) contours, hierarchy = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) for contour in contours: center, (width, height), angle = cv2.minAreaRect(contour) if -5 <= (width - height) <= 5 and 30 <= width <= 50: cv2.drawContours(img, [contour], -1, (0, 0, 255), 3) res.append(cv2.resize(img, (L, L))) resImg = np.zeros((L * N, L * N, 3), dtype=np.uint8) for i, img in enumerate(res): row, col = i // N, i % N x, y = col * L, row * L resImg[y:y + L, x:x + L] = img cv2.imshow("", resImg) cv2.waitKey(0) cv2.destroyAllWindows()
时间: 2023-12-15 10:05:52 浏览: 29
这是一段Python代码,主要的作用是对给定的maze.png图片进行处理并展示处理后的结果。具体包括以下步骤:
1. 导入cv2、glob和numpy模块。
2. 使用glob模块获取maze.png图片的路径,将其读取并调整大小为512x512。
3. 将图片转化为灰度图,并进行二值化处理。
4. 使用cv2.findContours函数找到图像中的所有轮廓,并选择面积最大的轮廓进行逼近处理。
5. 使用cv2.HoughCircles函数检测图像中的圆形,并在圆形周围画出红色圆圈。
6. 使用cv2.Canny函数进行边缘检测,再次使用cv2.findContours函数找到图像中的所有轮廓。
7. 对于每个轮廓,使用cv2.minAreaRect函数获取其最小外接矩形,并判断其长宽比是否在一定范围内,如果满足条件,则在轮廓周围画出红色矩形。
8. 将处理后的图片添加进一个列表中,并将所有图片通过numpy模块拼接成一个大图展示。
以上就是这段代码的大致执行过程。
相关问题
import glob import os import sys from pathlib import Path import cv2 as cv import numpy as np import scipy.io from PIL import Image
这是一段 Python 代码,其中导入了一些常用的库,包括:
- glob:用于查找符合特定规则的文件路径名。
- os:提供了许多与操作系统交互的函数。
- sys:提供了许多与 Python 解释器和运行时环境交互的函数。
- pathlib:提供了一种面向对象的路径操作方式。
- cv2:OpenCV 库,用于图像处理和计算机视觉。
- numpy:用于科学计算的 Python 库。
- scipy:用于数学、科学和工程计算的 Python 库。
- PIL:Python Imaging Library,用于图像处理和操作。
这些库可以用于各种各样的任务,例如图像处理、机器学习、数据分析等。
from tensorflow import keras import tensorflow as tf import matplotlib.pyplot as plt import numpy as np import glob意思
这段代码是用Python编写的,它导入了一些常用的机器学习和数据处理库,包括TensorFlow、Keras、Matplotlib和NumPy。
`from tensorflow import keras`:这行代码导入了Keras库,Keras是一个高级神经网络API,它可以用于构建和训练深度学习模型。
`import tensorflow as tf`:这行代码导入了TensorFlow库,TensorFlow是一个流行的开源机器学习框架,它提供了丰富的工具和功能,用于构建和训练机器学习模型。
`import matplotlib.pyplot as plt`:这行代码导入了Matplotlib库中的pyplot模块,它提供了绘制图表和可视化数据的功能。
`import numpy as np`:这行代码导入了NumPy库,NumPy是一个用于科学计算的Python库,它提供了多维数组对象和各种数学函数。
`import glob`:这行代码导入了Python标准库中的glob模块,它用于查找文件路径名匹配的文件。在这段代码中,可能会用到glob来查找文件路径。
请注意,代码片段中的具体用法和目的可能需要更多的上下文才能确定。如果你有更详细的代码或问题,请提供更多信息,我将尽力帮助你。
相关推荐
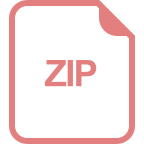













