禁忌搜索算法的原理及流程,并用python写一段禁忌搜索算法的代码
时间: 2024-03-18 18:40:11 浏览: 9
禁忌搜索算法是一种基于局部搜索的优化算法,在解空间中搜索最优解。它通过维护一个禁忌表来避免搜索过程中陷入局部最优解,从而在更广阔的解空间中寻找全局最优解。其基本流程如下:
1. 初始化初始解,并将其加入禁忌表;
2. 在邻域中搜索最优解,并检查是否在禁忌表中;
3. 如果不在禁忌表中,则更新当前解,并将其加入禁忌表;
4. 如果在禁忌表中,则重新在邻域中搜索,直到找到不在禁忌表中的最优解;
5. 更新禁忌表;
6. 如果满足终止条件,则输出最优解;否则回到第二步。
下面是使用Python实现禁忌搜索算法的代码示例:
```python
import random
# 定义目标函数
def target_func(x):
return x**2
# 定义禁忌搜索算法函数
def taboo_search(init_x, max_iter, tabu_length):
# 初始化当前解和最优解
cur_x = init_x
best_x = init_x
# 初始化禁忌表
tabu_list = [init_x]
# 迭代搜索
for i in range(max_iter):
# 在邻域中搜索最优解
neighbor_x = cur_x + random.uniform(-1, 1)
while neighbor_x in tabu_list:
neighbor_x = cur_x + random.uniform(-1, 1)
# 更新当前解和最优解
if target_func(neighbor_x) < target_func(cur_x):
cur_x = neighbor_x
if target_func(cur_x) < target_func(best_x):
best_x = cur_x
# 更新禁忌表
tabu_list.append(neighbor_x)
if len(tabu_list) > tabu_length:
tabu_list.pop(0)
# 返回最优解
return best_x
# 测试禁忌搜索算法函数
init_x = 5
max_iter = 100
tabu_length = 5
best_x = taboo_search(init_x, max_iter, tabu_length)
print("最优解为:", best_x)
print("最优解对应的函数值为:", target_func(best_x))
```
在上面的代码中,我们定义了一个简单的目标函数`target_func`,并使用禁忌搜索算法寻找该函数的最小值。我们设定初始解为5,最大迭代次数为100,禁忌表长度为5,最终输出禁忌搜索得到的最优解及其对应的函数值。
相关推荐
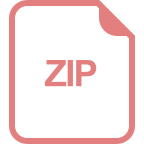
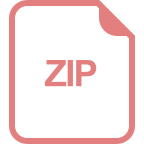














