c语言输入年和月,打印该年月有多少天
时间: 2024-10-10 11:05:18 浏览: 7
在C语言中,计算某个月份的天数通常需要考虑月份是否是闰年以及月份本身的天数规则。我们可以编写一个函数来实现这个功能,下面是一个简单的例子,它会根据给定的年份和月份判断该月有多少天:
```c
#include <stdio.h>
#include <stdbool.h>
// 判断是否是闰年的辅助函数
bool is_leap_year(int year) {
if (year % 4 == 0) {
if (year % 100 == 0) {
return year % 400 == 0;
}
return true;
}
return false;
}
// 计算指定年份和月份的天数
int days_in_month(int year, int month) {
switch(month) {
case 1: // January
case 3: // March
case 5: // May
case 7: // July
case 8: // August
case 10: // October
case 12: // December
return 31;
case 4: // April
case 6: // June
case 9: // September
case 11: // November
return 30;
case 2: // February
if (is_leap_year(year)) {
return 29;
} else {
return 28;
}
default:
return -1; // 非法月份,返回错误代码
}
}
int main() {
int year, month;
printf("请输入年份: ");
scanf("%d", &year);
printf("请输入月份(1-12): ");
scanf("%d", &month);
// 确保输入的是合法的月份
if (month >= 1 && month <= 12) {
int days = days_in_month(year, month);
if (days != -1) {
printf("该年的%2d月有%d天。\n", month, days);
} else {
printf("非法月份输入!\n");
}
} else {
printf("请输入有效的月份范围(1-12)\n");
}
return 0;
}
```
在这个程序里,我们首先检查是否是闰年,然后根据月份的不同给出对应的天数。如果输入的月份超出范围,我们会输出错误信息。
相关推荐
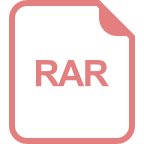
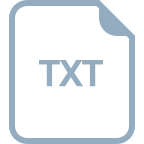
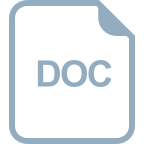














