用邻接表存储一个无向图,设计一个算法来统计度为2的顶点的个数
时间: 2024-12-08 08:16:49 浏览: 26
当使用邻接表(Adjacency List)来存储无向图时,每个顶点通常有一个链表,链表中的元素指向与该顶点相连的所有其他顶点。对于无向图,每条边连接两个顶点,所以如果一个顶点的入度(即连接它的边的数量)是2,这意味着从这个顶点出发有两条边分别指向其他两个顶点。
要计算度为2的顶点数量,我们可以遍历整个图,对于每个顶点,检查其相邻顶点列表的长度。如果长度恰好是2,说明当前顶点的度是2。下面是使用C语言实现的一个简单算法:
```c
#include <stdio.h>
#include <stdlib.h>
// 图节点结构体
typedef struct Node {
int vertex;
struct Node* next;
} Node;
// 创建邻接表表示图
void add_edge(Node** graph, int src, int dest) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->vertex = dest;
newNode->next = NULL;
if (*graph == NULL) {
*graph = newNode;
} else {
Node* temp = *graph;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 计算度为2的顶点数量
int count_degree_two(Node* graph, int V) {
int degree Two_count = 0;
for (int i = 0; i < V; i++) {
Node* current = graph[i];
if (current != NULL) {
int neighbors = 0;
Node* neighbor = current->next;
while (neighbor != NULL) {
neighbors++;
neighbor = neighbor->next;
}
if (neighbors == 2) {
degree_Two_count++;
}
}
}
return degree_Two_count;
}
int main() {
int V, E;
// 输入顶点数和边数
printf("Enter the number of vertices and edges: ");
scanf("%d %d", &V, &E);
// 初始化邻接表
Node** graph = (Node**)malloc(V * sizeof(Node*));
for (int i = 0; i < V; i++) {
graph[i] = NULL;
}
// 添加边
for (int i = 0; i < E; i++) {
int src, dest;
printf("Enter edge %d: from %d to %d\n", i+1, src, dest);
add_edge(&graph[src], src, dest); // 注意交换src和dest,因为无向图中每条边双向
}
int degreeTwoVertices = count_degree_two(graph, V);
printf("Number of vertices with degree 2: %d\n", degreeTwoVertices);
return 0;
}
```
在这个程序中,我们首先创建了一个邻接表表示图,然后定义了一个`count_degree_two`函数来计算度为2的顶点数量。用户可以通过`main`函数输入顶点和边的数量以及边的具体信息。
阅读全文
相关推荐
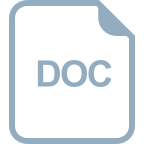
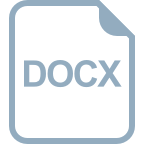
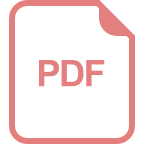















