写出将一个无向图的邻接表转换成邻接短阵的算法,请使用类c语法。
时间: 2023-12-09 21:01:39 浏览: 78
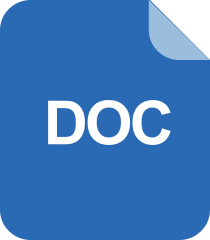
将一个无向图的邻接表转换为邻接矩阵算法.doc.doc

将一个无向图的邻接表转换成邻接短阵的算法可以如下实现:
首先,我们创建一个二维数组邻接矩阵adjMatrix,初始化所有元素为0。
然后,我们遍历邻接表,对于每一个顶点v,遍历其对应的邻接链表,得到其每一个邻接顶点w。
对于每个邻接顶点w,将邻接矩阵中坐标为(v,w)和(w,v)的元素设置为1,表示v和w之间存在边。
最后,遍历邻接矩阵,打印出每个元素即可。
下面是对应的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 100 // 最大顶点数
// 邻接表中的节点
typedef struct AdjListNode {
int dest;
struct AdjListNode* next;
} AdjListNode;
// 表示邻接表的头节点的结构体
typedef struct AdjList {
struct AdjListNode* head;
} AdjList;
// 表示图的结构体
typedef struct Graph {
int numVertices;
struct AdjList* array;
} Graph;
// 创建含有V个顶点的图
Graph* createGraph(int numVertices) {
Graph* graph = (Graph*)malloc(sizeof(Graph));
graph->numVertices = numVertices;
// 创建邻接表数组
graph->array = (AdjList*)malloc(numVertices * sizeof(AdjList));
// 将邻接表的头指针初始化为NULL
for (int i = 0; i < numVertices; ++i) {
graph->array[i].head = NULL;
}
return graph;
}
// 添加边到无向图中
void addEdge(Graph* graph, int src, int dest) {
// 添加一条从src到dest的边
AdjListNode* newNode = (AdjListNode*)malloc(sizeof(AdjListNode));
newNode->dest = dest;
newNode->next = graph->array[src].head;
graph->array[src].head = newNode;
// 添加一条从dest到src的边(因为是无向图)
newNode = (AdjListNode*)malloc(sizeof(AdjListNode));
newNode->dest = src;
newNode->next = graph->array[dest].head;
graph->array[dest].head = newNode;
}
// 将邻接表转换成邻接矩阵
void convertToAdjMatrix(Graph* graph) {
int numVertices = graph->numVertices;
int adjMatrix[numVertices][numVertices]; // 定义邻接矩阵
// 初始化邻接矩阵元素为0
for (int i = 0; i < numVertices; ++i) {
for (int j = 0; j < numVertices; ++j) {
adjMatrix[i][j] = 0;
}
}
// 遍历邻接表,转换成邻接矩阵
for (int v = 0; v < numVertices; ++v) {
AdjListNode* pCrawl = graph->array[v].head;
while (pCrawl) {
int w = pCrawl->dest;
adjMatrix[v][w] = 1;
pCrawl = pCrawl->next;
}
}
// 打印邻接矩阵
for (int i = 0; i < numVertices; ++i) {
for (int j = 0; j < numVertices; ++j) {
printf("%d ", adjMatrix[i][j]);
}
printf("\n");
}
}
int main() {
int numVertices = 5; // 图中顶点的个数
Graph* graph = createGraph(numVertices);
// 添加边
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
// 将邻接表转换成邻接矩阵
convertToAdjMatrix(graph);
return 0;
}
```
以上算法可以将一个无向图的邻接表转换成邻接短阵。
阅读全文
相关推荐
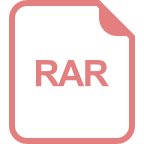
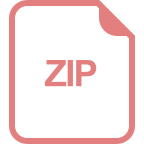
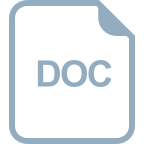
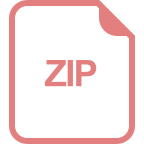
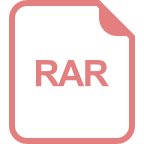
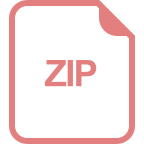
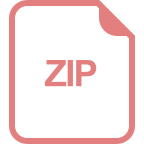
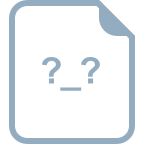
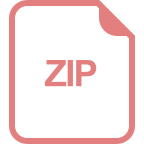
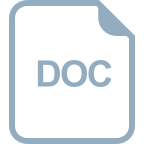
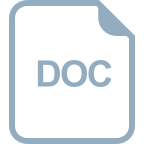
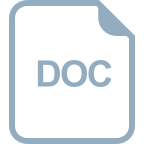
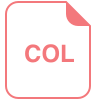
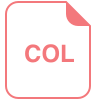
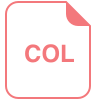
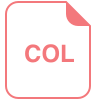