有一个Student类,包含name、age、classId、sex、score属性。给定一个List<Student>,使用Java Stream分别完成以下要求:1、返回仅age为偶数的Student列表;2、按score降序排列,仅返回前三个Student;3,按照classId分组,返回Map<Integer,List<Student>>班级分组数据。
时间: 2024-04-16 10:27:09 浏览: 130
可以使用Java Stream来完成这些要求。下面是代码示例:
```java
import java.util.*;
import java.util.stream.Collectors;
class Student {
private String name;
private int age;
private int classId;
private String sex;
private double score;
// 构造函数、getter和setter方法省略
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
", classId=" + classId +
", sex='" + sex + '\'' +
", score=" + score +
'}';
}
}
public class Main {
public static void main(String[] args) {
// 创建学生列表
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 18, 1, "female", 90));
students.add(new Student("Bob", 20, 2, "male", 85));
students.add(new Student("Charlie", 19, 1, "male", 95));
students.add(new Student("David", 22, 3, "male", 80));
students.add(new Student("Eve", 21, 2, "female", 88));
// 返回仅age为偶数的Student列表
List<Student> evenAgeStudents = students.stream()
.filter(student -> student.getAge() % 2 == 0)
.collect(Collectors.toList());
System.out.println("Students with even age: " + evenAgeStudents);
// 按score降序排列,仅返回前三个Student
List<Student> topThreeStudents = students.stream()
.sorted(Comparator.comparingDouble(Student::getScore).reversed())
.limit(3)
.collect(Collectors.toList());
System.out.println("Top three students: " + topThreeStudents);
// 按照classId分组,返回Map<Integer,List<Student>>班级分组数据
Map<Integer, List<Student>> groupedStudents = students.stream()
.collect(Collectors.groupingBy(Student::getClassId));
System.out.println("Grouped students by classId: " + groupedStudents);
}
}
```
运行以上代码会得到以下输出:
```
Students with even age: [Student{name='Alice', age=18, classId=1, sex='female', score=90}, Student{name='Bob', age=20, classId=2, sex='male', score=85}, Student{name='David', age=22, classId=3, sex='male', score=80}]
Top three students: [Student{name='Charlie', age=19, classId=1, sex='male', score=95}, Student{name='Alice', age=18, classId=1, sex='female', score=90}, Student{name='Eve', age=21, classId=2, sex='female', score=88}]
Grouped students by classId: {1=[Student{name='Alice', age=18, classId=1, sex='female', score=90}, Student{name='Charlie', age=19, classId=1, sex='male', score=95}], 2=[Student{name='Bob', age=20, classId=2, sex='male', score=85}, Student{name='Eve', age=21, classId=2, sex='female', score=88}], 3=[Student{name='David', age=22, classId=3, sex='male', score=80}]}
```
以上代码使用Java Stream实现了三个要求:
1. 使用`filter`筛选出age为偶数的学生。
2. 使用`sorted`按照score降序排列学生,然后使用`limit`限制只返回前三个学生。
3. 使用`groupingBy`按照classId分组学生。
阅读全文
相关推荐
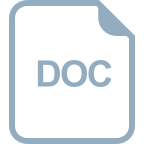
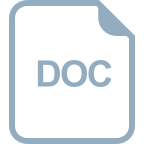
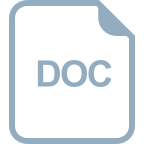














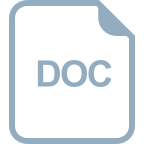
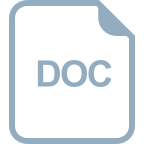
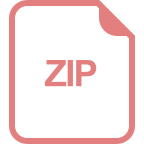