给出平面上a,b,c,d四个点的坐标,依次连接a-b,b-c,c-d,d-a,请你写c++程序判断得到的图形是否是凸四边形.
时间: 2024-10-13 20:06:16 浏览: 51
在C++中,判断一个由四条线段组成的图形是否是凸四边形通常涉及到向量运算和角度比较。以下是一个简单的算法描述和示例程序:
首先,你需要定义一个点类,包含x和y坐标,并提供必要的操作如加法、减法等。
```cpp
#include <iostream>
#include <cmath>
class Point {
public:
double x, y;
Point(double x = 0, double y = 0) : x(x), y(y) {}
Point operator+(const Point &other) { return Point(x + other.x, y + other.y); }
Point operator-(const Point &other) { return Point(x - other.x, y - other.y); }
};
// 计算两个点之间的向量
Point vector(const Point &p1, const Point &p2) {
return p2 - p1;
}
// 判断三点是否构成逆时针方向
bool isCounterClockwise(Point a, Point b, Point c) {
Point vec1 = vector(b, a);
Point vec2 = vector(c, a);
return crossProduct(vec1, vec2) > 0; // 如果叉积大于零,表示顺时针方向,反之则逆时针
}
// 凸四边形判定函数
bool isConvexPolygon(Point a, Point b, Point c, Point d) {
return isCounterClockwise(a, b, c) && isCounterClockwise(c, d, a); // 两对相邻边顺时针,则整个四边形是凸的
}
int main() {
Point points[] = { {x1, y1}, {x2, y2}, {x3, y3}, {x4, y4} }; // 假设已知四个点的坐标
if (isConvexPolygon(points[0], points[1], points[2], points[3])) {
std::cout << "The polygon is convex.\n";
} else {
std::cout << "The polygon is not convex.\n";
}
return 0;
}
// 叉积计算
double crossProduct(const Point &vec1, const Point &vec2) {
return vec1.x * vec2.y - vec1.y * vec2.x;
}
```
阅读全文
相关推荐
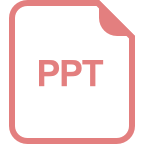
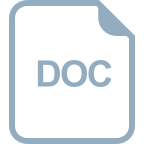
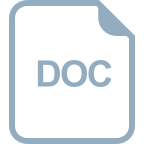


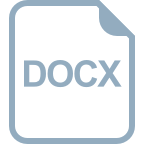



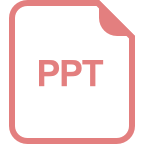
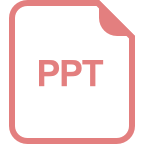
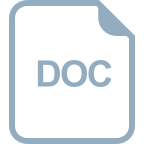
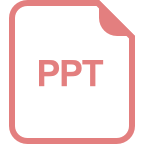



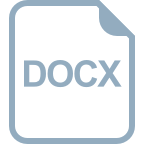
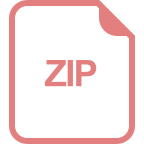